c++操作mongodb数据库,读取数据,并修改当前数据,增加新的列名。使用rapidjson
时间: 2024-09-21 08:11:26 浏览: 47
在C++中,使用RapidJSON库处理MongoDB数据库通常涉及到以下几个步骤:
1. **安装依赖**:
首先,你需要安装MongoDB C++驱动程序,例如`mongo-cxx-driver`,以及RapidJSON库。你可以从MongoDB官网下载对应版本的C++驱动。
2. **连接到MongoDB**:
使用`mongo::Client`类建立连接,提供服务器地址和端口信息。例如:
```cpp
mongo::Client client("mongodb://localhost:27017");
```
3. **选择数据库和集合**:
通过`client`对象选择你要操作的数据库和集合:
```cpp
mongo::Database db = client["your_database"];
mongo::Collection<Document> collection = db["your_collection"];
```
4. **读取数据**:
使用`find_one()`、`find()`等方法查询数据,比如:
```cpp
bsoncxx::document::view doc;
auto result = collection.find_one(filter);
if (result) {
doc = result->view();
// 处理查询结果
}
```
5. **修改数据**:
对读取到的数据进行更新,可以使用`update_one()`方法:
```cpp
bsoncxx::builder::document update;
update << "new_column_name" << value; // 新增的列值
collection.update_one(filter, update.view());
```
6. **添加新列**:
更新文档时直接插入新的键值对即可,上述代码中的`update`部分就是在做这个工作。
7. **处理JSON**:
如果你想用RapidJSON处理文档,首先需要将BSON文档转换成` rapidjson::Document`:
```cpp
rapidjson::Document jsonDoc;
bsoncxx::document::value_to_json(jsonDoc, doc);
```
8. **保存数据**:
修改后的`jsonDoc`可以直接序列化回MongoDB:
```cpp
bsoncxx::builder::document docBuilder;
rapidjson::Document::AllocatorType& allocator = jsonDoc.GetAllocator();
jsonDoc.Accept(docBuilder);
collection.replace_one(filter, docBuilder.view(), false);
```
相关推荐
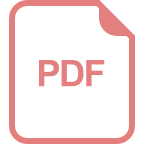
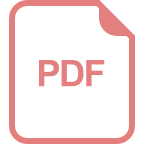
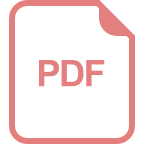














