python设计一个简单的购物系统
时间: 2024-09-14 15:14:28 浏览: 41
在Python中设计一个简单的购物系统可以通过创建几个类来实现,这些类将代表购物系统中的不同实体,例如商品、购物车和顾客等。下面是一个基本的设计思路:
1. 商品类(Product):用来表示购物系统中的商品,包含商品的基本信息,如商品名称、价格、库存数量等。
2. 购物车类(ShoppingCart):用来管理顾客所选择的商品,包括添加商品、删除商品、显示购物车中商品、计算总价等功能。
3. 顾客类(Customer):代表顾客,包含顾客的基本信息,如姓名、购物车等,还可以有其他如地址、支付方法等属性。
4. 购物系统类(ShoppingSystem):用来管理商品、顾客以及整个购物流程,如添加商品、处理顾客购买等。
下面是一个简单的示例代码:
```python
class Product:
def __init__(self, name, price, stock):
self.name = name
self.price = price
self.stock = stock
def __str__(self):
return f"{self.name} - ${self.price} (库存: {self.stock})"
class ShoppingCart:
def __init__(self):
self.products = []
def add_product(self, product, quantity):
if product.stock >= quantity:
product.stock -= quantity
self.products.append((product, quantity))
print(f"已添加 {quantity} 个 {product.name} 到购物车。")
else:
print(f"库存不足,无法添加 {quantity} 个 {product.name} 到购物车。")
def remove_product(self, product):
for item in self.products:
if item[0] == product:
product.stock += item[1]
self.products.remove(item)
print(f"{product.name} 已从购物车中移除。")
return
def calculate_total(self):
return sum([product[0].price * product[1] for product in self.products])
def show_cart(self):
for item in self.products:
product, quantity = item
print(f"{product.name} x {quantity} = ${product.price * quantity}")
print(f"总计: ${self.calculate_total()}")
class Customer:
def __init__(self, name):
self.name = name
self.shopping_cart = ShoppingCart()
class ShoppingSystem:
def __init__(self):
self.products = []
def add_product(self, product):
self.products.append(product)
def create_customer(self, name):
return Customer(name)
# 示例使用
system = ShoppingSystem()
product1 = Product("笔记本电脑", 5000, 10)
product2 = Product("手机", 3000, 20)
system.add_product(product1)
system.add_product(product2)
customer = system.create_customer("张三")
customer.shopping_cart.add_product(product1, 1)
customer.shopping_cart.add_product(product2, 2)
customer.shopping_cart.show_cart()
```
这个简单的购物系统可以根据需要进行扩展,比如添加支付功能、订单管理、用户登录等更多功能。
阅读全文
相关推荐
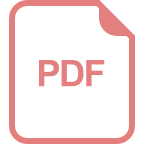
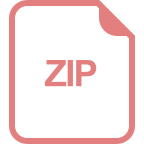
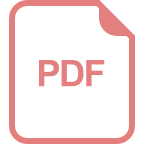


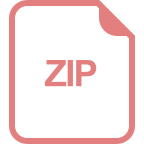
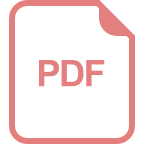
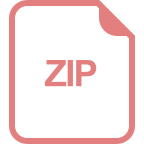
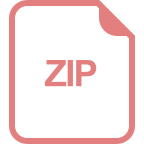
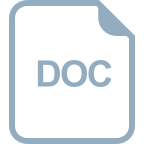
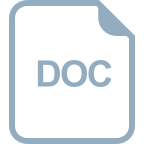
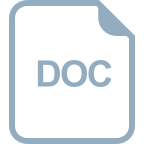
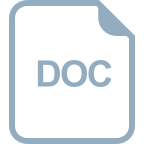
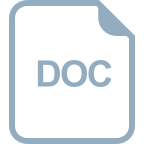
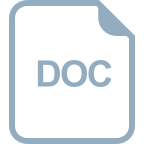
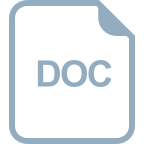



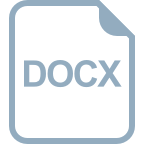