python 多线程 矩阵运算
时间: 2023-12-14 10:34:35 浏览: 215
在Python中,可以使用多线程来加速矩阵运算。下面是一个使用多线程进行矩阵向量乘法的示例代码:
```python
import numpy as np
import threading
# 定义线程类
class MatrixVectorThread(threading.Thread):
def __init__(self, matrix, vector, result, row):
threading.Thread.__init__(self)
self.matrix = matrix
self.vector = vector
self.result = result
self.row = row
def run(self):
# 计算矩阵的一行与向量的乘积
self.result[self.row] = np.dot(self.matrix[self.row], self.vector)
def parallel_matrix_vector_multiplication(matrix, vector):
# 创建结果数组
result = np.zeros(matrix.shape[0])
# 创建线程列表
threads = []
for i in range(matrix.shape[0]):
thread = MatrixVectorThread(matrix, vector, result, i)
thread.start()
threads.append(thread)
# 等待所有线程完成
for thread in threads:
thread.join()
return result
```
在这个示例代码中,我们首先定义了一个`MatrixVectorThread`类,用于计算矩阵的一行与向量的乘积。然后,我们定义了一个`parallel_matrix_vector_multiplication`函数,该函数使用多线程来计算矩阵与向量的乘积。具体来说,该函数首先创建一个结果数组,然后创建一个线程列表,每个线程计算矩阵的一行与向量的乘积,并将结果存储在结果数组中。最后,该函数等待所有线程完成,并返回结果数组。
阅读全文
相关推荐
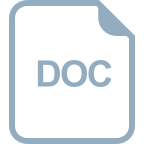
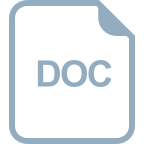
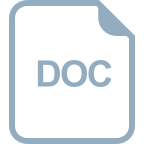
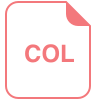
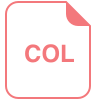
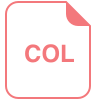

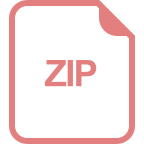
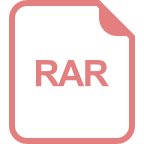
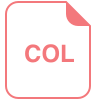
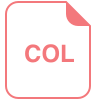
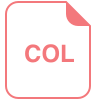
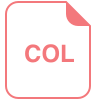
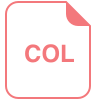
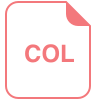
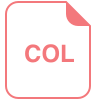
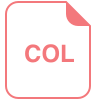
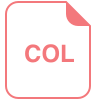
