设计一个网站注册页面,包含账号、姓名、年龄、手机号、密码,确认密码、邮箱、地址。
时间: 2024-09-15 19:03:45 浏览: 182
设计一个网站注册页面需要考虑用户体验和安全性,以下是基本的布局和元素说明:
**HTML结构**
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>网站注册</title>
<style>
/* CSS 样式可自定义 */
body {
font-family: Arial, sans-serif;
}
form {
width: 400px;
margin: auto;
padding: 20px;
border: 1px solid #ccc;
}
label, input, button {
display: block;
margin-bottom: 10px;
}
</style>
</head>
<body>
<h2>新用户注册</h2>
<form action="register.php" method="post" novalidate>
<div>
<label for="account">账号:</label>
<input type="text" id="account" name="account" required pattern="[a-zA-Z0-9._]{5,}" title="请输入5至20位字符的账号">
</div>
<div>
<label for="name">姓名:</label>
<input type="text" id="name" name="name" required>
</div>
<div>
<label for="age">年龄:</label>
<input type="number" id="age" name="age" min="18" max="120" required>
</div>
<div>
<label for="phone">手机号:</label>
<input type="tel" id="phone" name="phone" required pattern="\d{11}">
</div>
<div>
<label for="password">密码:</label>
<input type="password" id="password" name="password" required minlength="6" maxlength="20">
<small>(密码长度6-20位)</small>
</div>
<div>
<label for="confirm_password">确认密码:</label>
<input type="password" id="confirm_password" name="confirm_password" required equalTo="#password">
<small>(两次输入密码需一致)</small>
</div>
<div>
<label for="email">邮箱:</label>
<input type="email" id="email" name="email" required>
</div>
<div>
<label for="address">地址:</label>
<textarea id="address" name="address" rows="4" cols="30" required></textarea>
</div>
<button type="submit">注册</button>
<p class="error" style="display:none;"></p>
</form>
</body>
</html>
```
**PHP后端 (register.php)**:
(这里只是一个基础的处理,实际应用需要完整的错误检查和数据库操作)
```php
<?php
if ($_SERVER["REQUEST_METHOD"] === "POST") {
// 验证和存储用户数据
$data = [
'account' => trim($_POST['account']),
// ...其他字段类似...
];
// 验证数据(如邮箱格式、手机号合法性等)
if (validateData($data)) {
// 连接数据库并插入数据
insertIntoDatabase($data);
echo "注册成功!";
} else {
echo "注册失败,请检查填写的信息。";
}
}
function validateData($data) {
// 实现具体的验证逻辑
// ...
}
function insertIntoDatabase($data) {
// 这里只是模拟,实际操作需要连接数据库并添加适当的错误处理
// ...
}
?>
```
这个例子展示了注册页面的基本构成和一些简单的验证规则。在实际项目中,还要考虑使用HTTPS、验证码以及更强的身份验证策略,同时遵守相关的隐私法规。
阅读全文
相关推荐
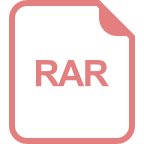
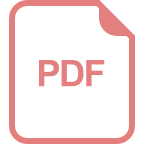
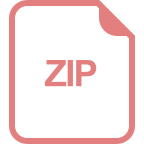
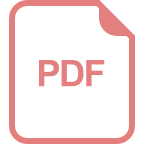
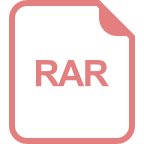
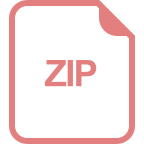
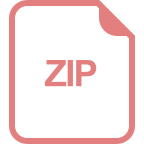
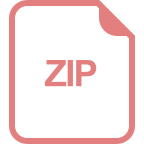
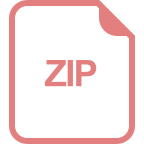
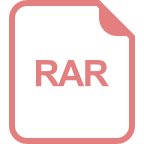
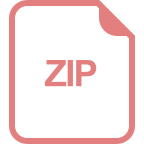
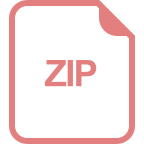
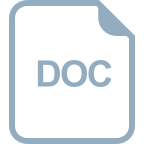



