在Vue中,如何使用Element UI的el-table组件以及type='expand'属性来创建一个具有父子级数据结构的表格,并同时确保子表格也支持分页功能?请提供相应的代码示例。
时间: 2024-11-25 21:32:54 浏览: 6
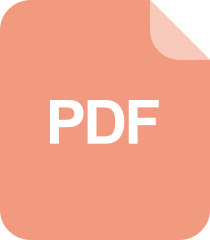
vue2.0 + element UI 中 el-table 数据导出Excel的方法
在Vue中,你可以使用Element UI的`el-table`组件结合`type='expand'`属性来创建一个具有父子级数据结构的表格。同时,为了实现子表格的分页功能,可以利用Element UI的`el-pagination`组件配合`v-for`循环来达到目的。下面是一个基本的代码示例:
首先,你需要安装Element UI和Vue CLI,然后在你的项目中引入Element UI:
```bash
npm install element-ui vue
```
在main.js文件中注册Element UI:
```javascript
import Vue from 'vue'
import ElementUI from 'element-ui'
import 'element-ui/lib/theme-chalk/index.css'
Vue.use(ElementUI)
```
接下来,在你的组件中,假设你有一个数据模型`data`,它包含父子层级的数据,比如:
```javascript
export default {
data() {
return {
data: [
{
id: 1,
name: '父级一',
children: [
{ id: 11, name: '子级11', },
{ id: 12, name: '子级12', },
]
},
// 更多数据...
],
currentPage: 1, // 当前页码
pageSize: 5, // 每页显示的条数
}
},
}
```
然后在模板部分,创建`el-table`和`el-pagination`:
```html
<template>
<div>
<el-table :data="data" style="width: 100%">
<el-table-column prop="name" label="名称"></el-table-column>
<el-table-column type="expand">
<template #default="{ row }">
<el-table :data="row.children.slice((currentPage - 1) * pageSize, currentPage * pageSize)" border></el-table>
<el-pagination
@current-change="handleCurrentChange"
:page-size="pageSize"
:total="getChildrenLength(row)"
></el-pagination>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
// ... 上述data部分
methods: {
handleCurrentChange(page) {
this.currentPage = page;
// 更新子表格数据
this.updateChildData();
},
getChildrenLength(row) {
return row.children.length;
},
updateChildData() {
// 这里可以根据当前页码和每页大小计算并更新子级数据,例如:row.children.slice(this.currentPage * this.pageSize - this.pageSize, this.currentPage * this.pageSize);
},
},
</script>
```
在这个例子中,`handleCurrentChange`方法会在用户点击分页按钮时触发,更新子表格的内容。`updateChildData`方法用于获取子表格实际需要的数据。
注意这只是一个基础示例,实际应用中可能需要处理更多的边界情况和性能优化。例如,如果数据量非常大,可能需要考虑懒加载策略来提高性能。
阅读全文
相关推荐
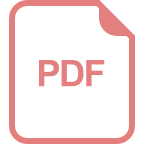
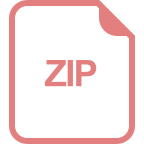
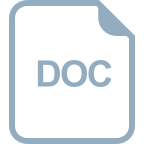
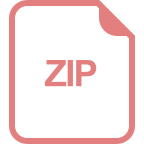
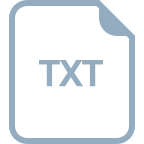
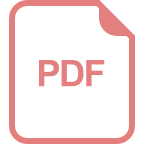
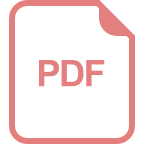
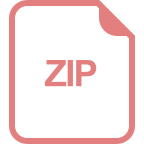
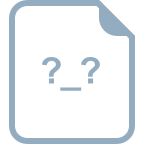
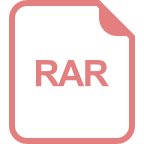
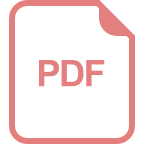
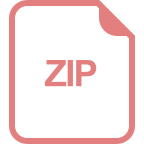
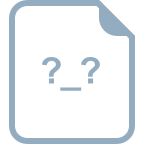
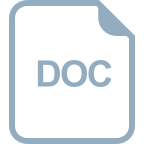
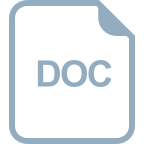
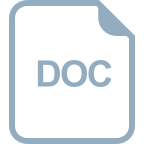