1.实现目录创建操作(程序代码) 2.实现文件上传操作(程序代码) 3.实现文件列表查看(程序代码) 4.实现目录删除操作(程序代码) hadoop
时间: 2024-09-28 15:09:21 浏览: 5
在Hadoop框架中,以下是使用Java API实现一些基本文件系统操作的简单示例,这里假设我们已经有一个Hadoop配置环境:
1. **实现目录创建操作**:
```java
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.conf.Configuration;
public void createDirectory(String path) throws IOException {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
fs.mkdirs(new Path(path));
System.out.println("Directory created at " + path);
}
```
2. **实现文件上传操作**:
```java
public void uploadFile(String srcPath, String dstPath) throws IOException {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
fs.copyFromLocalFile(new Path(srcPath), new Path(dstPath));
System.out.println("File uploaded from " + srcPath + " to " + dstPath);
}
```
3. **实现文件列表查看**:
```java
public void listFiles(String directoryPath) throws IOException {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
FileStatus[] fileStatuses = fs.listStatus(new Path(directoryPath));
if (fileStatuses != null) {
for (FileStatus status : fileStatuses) {
System.out.println(status.getPath().getName());
}
} else {
System.out.println("Directory is empty or not found.");
}
}
```
4. **实现目录删除操作**:
```java
public void deleteDirectory(String path) throws IOException {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
boolean result = fs.delete(new Path(path), true); // Recursive deletion with true parameter
if (result) {
System.out.println("Directory deleted at " + path);
} else {
System.out.println("Failed to delete directory or does not exist.");
}
}
```
注意:这只是一个基础的示例,实际应用中可能需要处理异常并确保用户有相应的权限。同时,在Hadoop分布式环境下,文件操作通常会在HDFS上进行,而不仅仅是本地文件系统。
相关推荐
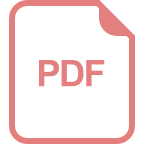
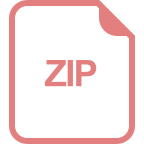
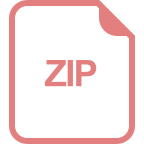
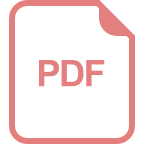
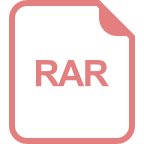
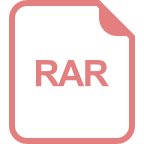
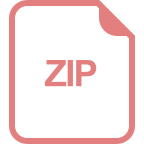
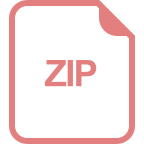
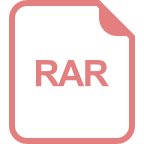
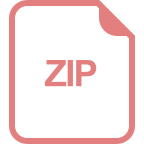
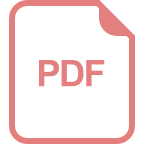
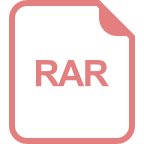
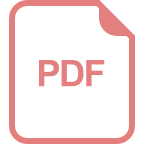
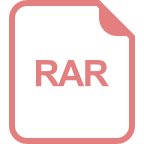
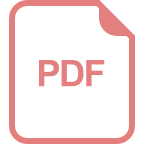
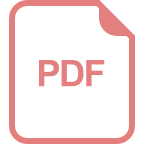
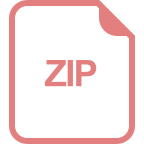
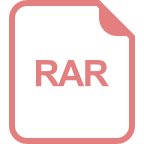