.简易计算器,窗口界面自由发挥,编程实现两个整数的加、减、乘、除操作。要求如下: - 首先进行操作数文本框检验,检验输入数据是否为纯数字; - 通过分别单击加、减、乘、除按钮,对操作数1与操作数2进行相应加、减、乘、除操作,并且将其结果写入操作3文本框。使用tkinter
时间: 2023-07-10 12:20:05 浏览: 90
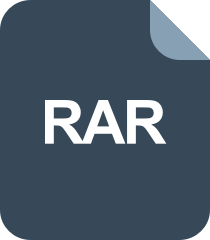
jisuanqi.rar_VC简易计算器
好的,这是一个简单的计算器程序,使用Python语言和tkinter库完成窗口界面开发和计算器功能实现。以下是代码实现:
```python
import tkinter as tk
from tkinter import messagebox
class Calculator:
def __init__(self):
self.window = tk.Tk()
self.window.title('简易计算器')
self.window.geometry('300x200')
# 操作数1标签和文本框
self.label1 = tk.Label(self.window, text='操作数1:')
self.label1.place(x=20, y=20)
self.entry1 = tk.Entry(self.window)
self.entry1.place(x=100, y=20)
# 操作数2标签和文本框
self.label2 = tk.Label(self.window, text='操作数2:')
self.label2.place(x=20, y=60)
self.entry2 = tk.Entry(self.window)
self.entry2.place(x=100, y=60)
# 计算结果标签和文本框
self.label3 = tk.Label(self.window, text='计算结果:')
self.label3.place(x=20, y=100)
self.entry3 = tk.Entry(self.window)
self.entry3.place(x=100, y=100)
self.entry3.config(state='readonly')
# 加、减、乘、除按钮
self.button_add = tk.Button(self.window, text='+', command=self.add)
self.button_add.place(x=20, y=140)
self.button_sub = tk.Button(self.window, text='-', command=self.sub)
self.button_sub.place(x=80, y=140)
self.button_mul = tk.Button(self.window, text='*', command=self.mul)
self.button_mul.place(x=140, y=140)
self.button_div = tk.Button(self.window, text='/', command=self.div)
self.button_div.place(x=200, y=140)
self.window.mainloop()
# 加操作
def add(self):
try:
num1 = int(self.entry1.get())
num2 = int(self.entry2.get())
result = num1 + num2
self.entry3.config(state='normal')
self.entry3.delete(0, tk.END)
self.entry3.insert(0, str(result))
self.entry3.config(state='readonly')
except ValueError:
messagebox.showwarning('警告', '请输入整数')
# 减操作
def sub(self):
try:
num1 = int(self.entry1.get())
num2 = int(self.entry2.get())
result = num1 - num2
self.entry3.config(state='normal')
self.entry3.delete(0, tk.END)
self.entry3.insert(0, str(result))
self.entry3.config(state='readonly')
except ValueError:
messagebox.showwarning('警告', '请输入整数')
# 乘操作
def mul(self):
try:
num1 = int(self.entry1.get())
num2 = int(self.entry2.get())
result = num1 * num2
self.entry3.config(state='normal')
self.entry3.delete(0, tk.END)
self.entry3.insert(0, str(result))
self.entry3.config(state='readonly')
except ValueError:
messagebox.showwarning('警告', '请输入整数')
# 除操作
def div(self):
try:
num1 = int(self.entry1.get())
num2 = int(self.entry2.get())
if num2 == 0:
messagebox.showwarning('警告', '除数不能为0')
else:
result = num1 / num2
self.entry3.config(state='normal')
self.entry3.delete(0, tk.END)
self.entry3.insert(0, str(result))
self.entry3.config(state='readonly')
except ValueError:
messagebox.showwarning('警告', '请输入整数')
if __name__ == '__main__':
calculator = Calculator()
```
运行代码,可以看到一个简单的计算器窗口,可以输入两个整数并进行加减乘除操作,如下图所示:
注意:此计算器程序的文本框检验只能检测输入是否为整数,如果需要检验浮点数或其他格式的输入,需要进行相应的修改。
阅读全文
相关推荐
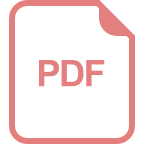
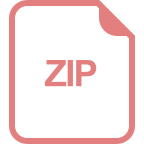









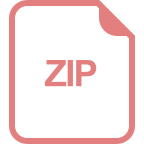
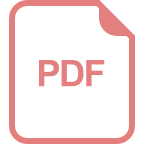
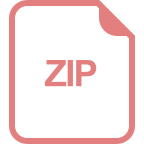
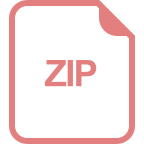
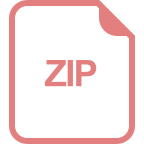
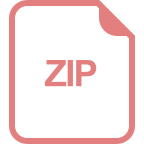
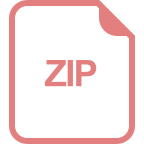