指出下列程序的错误,说明错误的原因并改正。 #include <iostream> using namespace std; class Student { string name; float score; static int total; int Student (const char n, float s) { name = n; total += s; return 0; } }; int main() { int Student::total = 0; Student s1("张三", 95); Student s2("李四", 90); Student s3("王五", 85); cout << "总分=" << total << endl; }
时间: 2024-01-28 08:03:03 浏览: 117
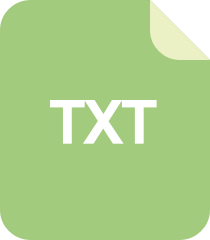
#include_iostream.h_

这个程序有以下错误:
1. 在 class Student 中的构造函数应该是 public,而不是 private。
2. 构造函数的参数类型应该是 const char* 而不是 const char。
3. 在构造函数中,total += s 应该改为 score = s; total += s;。
4. 在 main 函数中,应该先声明并初始化静态变量 total,然后才能创建对象。
5. 在 main 函数中,输出总分时应该使用类名 Student::total 而不是 total。
下面是改正后的程序:
```
#include <iostream>
#include <string>
using namespace std;
class Student {
string name;
float score;
static int total;
public:
Student(const char* n, float s) {
name = n;
score = s;
total += s;
}
};
int Student::total = 0;
int main() {
Student s1("张三", 95);
Student s2("李四", 90);
Student s3("王五", 85);
cout << "总分=" << Student::total << endl;
return 0;
}
```
改正后的程序中,构造函数被声明为 public,参数类型改为 const char*,在构造函数中同时给 name 和 score 赋值,先声明并初始化静态变量 total,然后才能创建对象,输出总分时使用类名 Student::total。
阅读全文
相关推荐
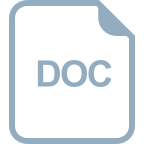
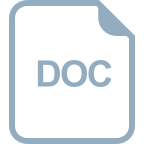









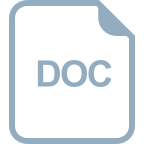
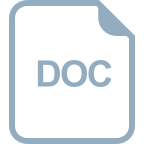
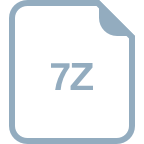
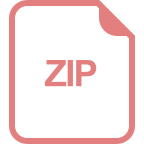
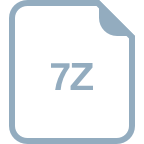