用c语言实现两个瓶子A和B,分别盛放醋和酱油,要求将它们互換(即A瓶原来盛醋,现改盛酱油,B瓶则相反
时间: 2024-10-13 12:14:30 浏览: 25
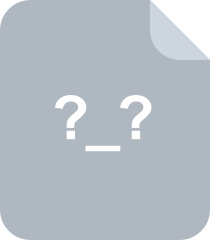
C语言程序设计-求两个整数a与b之和
在C语言中,我们可以使用指针来实现这个简单的容器交换操作。假设我们已经有一个结构体`Container`,它有两个成员变量`name`表示液体类型(例如,`type`为`Vinegar`或`SoySauce`),以及`quantity`表示容量。以下是如何使用指针来完成瓶子互换:
```c
#include <stdio.h>
typedef enum { Vinegar, SoySauce } LiquidType;
// 容器结构体
struct Container {
LiquidType type;
float quantity;
};
// 交换容器中的液体类型
void swapContainers(struct Container* a, struct Container* b) {
// 使用临时变量保存一个容器的数据
struct Container temp = *a;
// 将另一个容器的内容赋给第一个容器
*a = *b;
// 再将临时变量的内容赋给第二个容器
*b = temp;
}
int main() {
// 初始化两个容器
struct Container bottleA = {Vinegar, 50.0};
struct Container bottleB = {SoySauce, 75.0};
printf("Before swapping:\n");
printf("A: %s (%.1f ml)\n", bottleA.type == Vinegar ? "Vinegar" : "Soy Sauce", bottleA.quantity);
printf("B: %s (%.1f ml)\n", bottleB.type == SoySauce ? "Soy Sauce" : "Vinegar", bottleB.quantity);
// 交换瓶子内容
swapContainers(&bottleA, &bottleB);
printf("\nAfter swapping:\n");
printf("A: %s (%.1f ml)\n", bottleA.type == Vinegar ? "Vinegar" : "Soy Sauce", bottleA.quantity);
printf("B: %s (%.1f ml)\n", bottleB.type == SoySauce ? "Soy Sauce" : "Vinegar", bottleB.quantity);
return 0;
}
```
阅读全文
相关推荐
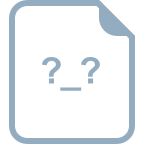
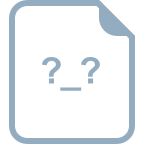















