本题要求用c语言编写程序,输入10个字符,统计其中英文字母、空格或回车、数字字符和其他字符的个数。
时间: 2023-08-31 19:20:22 浏览: 152
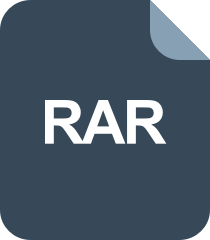
tj.rar_visual c_数字字符个数
### 回答1:
下面是一个用C语言编写的程序,可以输入10个字符,统计其中英文字母、空格或回车、数字字符和其他字符的个数:
```c
#include <stdio.h>
int main() {
int letters = 0, spaces = 0, digits = 0, others = 0;
char c;
printf("请输入10个字符:\n");
for (int i = 0; i < 10; i++) {
scanf("%c", &c);
if ((c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z')) {
letters++;
} else if (c == ' ' || c == '\n') {
spaces++;
} else if (c >= '0' && c <= '9') {
digits++;
} else {
others++;
}
}
printf("字母个数:%d\n", letters);
printf("空格或回车个数:%d\n", spaces);
printf("数字个数:%d\n", digits);
printf("其他字符个数:%d\n", others);
return 0;
}
```
这个程序中,定义了四个变量 `letters`、`spaces`、`digits` 和 `others`,用于记录不同类型字符的个数。在循环中,输入每个字符并根据其类型对相应的计数器进行增加。最后,输出各个类型字符的个数。
### 回答2:
这是一个简单的C语言程序,可以实现统计输入的10个字符中英文字母、空格或回车、数字字符和其他字符的个数。
```c
#include <stdio.h>
int main() {
char ch;
int letterCount = 0, spaceCount = 0, digitCount = 0, othersCount = 0;
printf("请输入10个字符:\n");
for (int i = 0; i < 10; ++i) {
scanf(" %c", &ch); // 注意空格,以避免读取之前的换行字符
if ((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) {
++letterCount;
} else if (ch == ' ' || ch == '\n') {
++spaceCount;
} else if (ch >= '0' && ch <= '9') {
++digitCount;
} else {
++othersCount;
}
}
printf("英文字母个数:%d\n", letterCount);
printf("空格或回车个数:%d\n", spaceCount);
printf("数字字符个数:%d\n", digitCount);
printf("其他字符个数:%d\n", othersCount);
return 0;
}
```
程序中定义了四个变量用于统计字符的个数。通过循环读取10个字符,并根据字符的范围判断它们属于哪一类字符。最后将统计结果打印出来。
### 回答3:
以下是用C语言编写的程序,用于输入10个字符并统计其中英文字母、空格或回车、数字字符和其他字符的个数:
```c
#include <stdio.h>
int main() {
char input[10];
int letters = 0, spacesOrReturns = 0, digits = 0, others = 0;
printf("请输入10个字符:\n");
for (int i = 0; i < 10; i++) {
scanf(" %c", &input[i]); // 输入的字符存储在input数组中
}
for (int i = 0; i < 10; i++) {
if ((input[i] >= 'a' && input[i] <= 'z') || (input[i] >= 'A' && input[i] <= 'Z')) {
letters++; // 统计英文字母的个数
} else if (input[i] == ' ' || input[i] == '\n') {
spacesOrReturns++; // 统计空格或回车的个数
} else if (input[i] >= '0' && input[i] <= '9') {
digits++; // 统计数字字符的个数
} else {
others++; // 统计其他字符的个数
}
}
printf("英文字母的个数:%d\n", letters);
printf("空格或回车的个数:%d\n", spacesOrReturns);
printf("数字字符的个数:%d\n", digits);
printf("其他字符的个数:%d\n", others);
return 0;
}
```
该程序首先会提示用户输入10个字符,然后使用循环将输入的字符依次存储到`input`数组中。接下来,通过遍历`input`数组,对每个字符进行判断和分类统计。统计完成后,程序会输出英文字母、空格或回车、数字字符和其他字符的个数。
请注意,上述程序假定输入的字符中只包括字母、空格或回车、数字字符和其他字符。如果有其他特殊字符或需求,可能需要对程序进行适当修改。
阅读全文
相关推荐
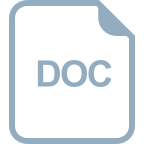
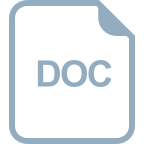










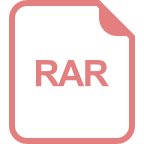




