import java.util.Scanner; public class Login { private static String username = "张三"; private static String password = "123456"; public static void main(String[] args) throws ToomanyErrorsException, NamePasswordMismatchException { Scanner scanner = new Scanner(System.in); int count = 0; System.out.println("请输入用户名: "); String username = scanner.next(); while (true) { System.out.println("请输入密码: "); String password = scanner.next(); if ("登录成功".equals(login(username, password))) { System.out.println("登录成功"); break; } count++; if (count==3){ throw new ToomanyErrorsException("密码输入次数已达上限!!!"); } } } public static String login(String username, String password) throws NamePasswordMismatchException { if (!Login.username.equals(username)) { System.out.println("该用户名尚未注册,请核对后输入"); return "用户名有误"; } else { if (!Login.password.equals(password)) { try { throw new NamePasswordMismatchException("密码输入有误"); } catch (Exception e) { e.printStackTrace(); } } else { return "登录成功"; } return "密码有误"; } } }哪里有问题
时间: 2023-12-19 22:04:04 浏览: 31
代码中存在以下问题:
1. 异常处理不恰当。在 `login` 方法中,当密码输入有误时,应该抛出 `NamePasswordMismatchException` 异常。但是,在异常处理代码中,只是简单地打印了堆栈信息,没有处理异常。应该将异常向上抛出,由 `main` 方法中的异常处理代码来处理。
2. 代码中的变量名冲突。在 `main` 方法中,定义了一个名为 `username` 的局部变量,与类中的静态变量 `username` 名称相同,会导致局部变量覆盖类中的静态变量。应该使用不同的变量名,或者使用 `this.username` 来引用类中的静态变量。
3. 没有处理用户名输入有误的情况。当输入的用户名与预设的用户名不一致时,应该提示用户该用户名尚未注册,并要求重新输入用户名。
4. 在 `login` 方法中,当密码输入有误时,返回的字符串为 "密码有误",但是实际上应该抛出 `NamePasswordMismatchException` 异常。
下面是修改过后的代码:
```java
import java.util.Scanner;
public class Login {
private static String username = "张三";
private static String password = "123456";
public static void main(String[] args) throws ToomanyErrorsException, NamePasswordMismatchException {
Scanner scanner = new Scanner(System.in);
int count = 0;
System.out.println("请输入用户名: ");
String inputUsername = scanner.next();
while (!username.equals(inputUsername)) {
System.out.println("该用户名尚未注册,请核对后输入");
System.out.println("请输入用户名: ");
inputUsername = scanner.next();
}
while (true) {
System.out.println("请输入密码: ");
String inputPassword = scanner.next();
if ("登录成功".equals(login(inputUsername, inputPassword))) {
System.out.println("登录成功");
break;
}
count++;
if (count == 3) {
throw new ToomanyErrorsException("密码输入次数已达上限!!!");
}
}
}
public static String login(String username, String password) throws NamePasswordMismatchException {
if (!Login.password.equals(password)) {
throw new NamePasswordMismatchException("密码输入有误");
} else {
return "登录成功";
}
}
}
```
相关推荐
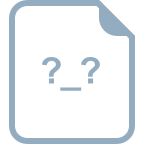







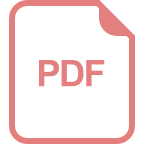
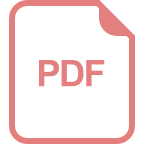
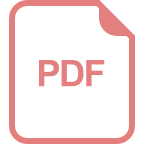
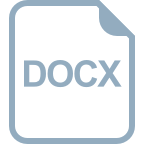
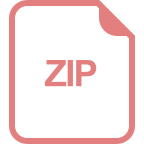
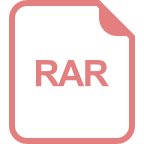