java调用https接口并跳过验证
时间: 2023-12-09 12:08:16 浏览: 147
在Java中调用HTTPS接口并跳过验证,可以通过创建自定义的TrustManager和HostnameVerifier来实现。
以下是一个示例代码:
```java
import java.security.SecureRandom;
import javax.net.ssl.*;
import java.net.URL;
import java.io.*;
public class HttpsClient {
public static void main(String[] args) throws Exception {
// 创建SSLContext对象
SSLContext sslContext = SSLContext.getInstance("SSL");
// 实现一个X509TrustManager接口,用于绕过验证,不用修改里面的方法
X509TrustManager trustManager = new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] chain, String authType) {}
public void checkServerTrusted(X509Certificate[] chain, String authType) {}
public X509Certificate[] getAcceptedIssuers() { return null; }
};
// 将X509TrustManager组合成TrustManager数组
TrustManager[] trustManagers = new TrustManager[] { trustManager };
// 初始化SSLContext
sslContext.init(null, trustManagers, new SecureRandom());
// 获取SSLSocketFactory对象
SSLSocketFactory sslSocketFactory = sslContext.getSocketFactory();
// 打开URL连接
URL url = new URL("https://example.com");
HttpsURLConnection conn = (HttpsURLConnection) url.openConnection();
// 设置连接属性
conn.setSSLSocketFactory(sslSocketFactory);
conn.setHostnameVerifier(new HostnameVerifier() {
public boolean verify(String hostname, SSLSession session) { return true; }
});
// 发送请求
InputStream input = conn.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(input));
StringBuilder response = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
System.out.println(response.toString());
// 关闭连接
conn.disconnect();
}
}
```
在上面的代码中,我们创建了一个自定义的TrustManager,该TrustManager不会执行任何验证操作。在初始化SSLContext时,我们将该TrustManager作为参数传递给init方法,从而实现绕过验证。
同时,我们还创建了一个自定义的HostnameVerifier,该HostnameVerifier始终返回true,从而实现跳过主机名验证。在设置HttpsURLConnection的连接属性时,我们将该HostnameVerifier作为参数传递给setHostnameVerifier方法。
阅读全文
相关推荐
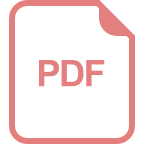



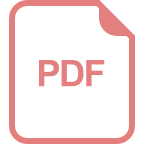
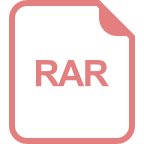
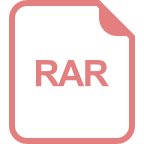









