linux下编写C语言程序删除指定目录下的空目录,要求不递归删除
时间: 2024-09-27 10:16:40 浏览: 37
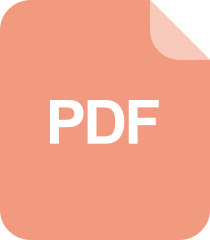
Linux_C编程一站式学习_最终版__带目录的pdf更方便查找与做笔记.pdf
在Linux环境下,如果你想要使用C语言编写程序来删除指定目录下的所有空目录,但不包括目录本身,可以利用`rmdir()`函数配合遍历目录结构来实现。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <sys/stat.h>
void delete_empty_subdirs(char *dir_path) {
struct dirent *dp;
DIR *dir = opendir(dir_path);
if (dir == NULL) {
perror("Error opening directory");
return;
}
while ((dp = readdir(dir)) != NULL) {
char subdir_path[PATH_MAX];
snprintf(subdir_path, PATH_MAX, "%s/%s", dir_path, dp->d_name);
struct stat st;
if (stat(subdir_path, &st) == 0 && S_ISDIR(st.st_mode)) { // 检查是否为目录
if (!S_ISDIR(st.st_nlink)) { // 如果链接数为1,说明目录下无内容
if (rmdir(subdir_path) == 0) {
printf("Deleted empty directory: %s\n", subdir_path);
} else {
perror("Error deleting directory");
}
} else {
printf("%s is not an empty directory.\n", subdir_path);
}
}
}
closedir(dir);
}
int main() {
char dir_to_clean[] = "/path/to/directory"; // 替换为你要清理的实际目录路径
delete_empty_subdirs(dir_to_clean);
return 0;
}
```
这个程序首先打开目标目录,然后遍历目录中的每个文件和目录。如果遇到的是一个空目录(即其链接数为1),则尝试使用`rmdir()`函数删除它。注意,`rmdir()`仅能删除空目录,所以只有当目录确实是空的,删除操作才会成功。
阅读全文
相关推荐
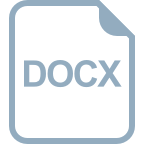
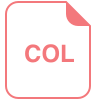
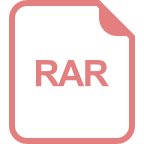
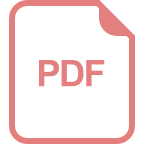
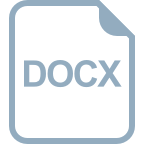
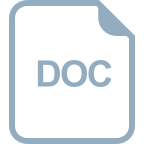
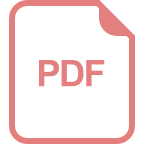
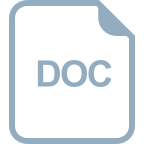
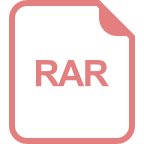
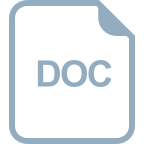
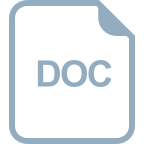
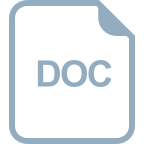
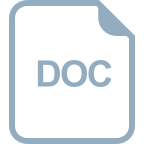
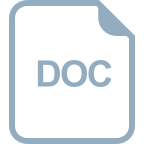
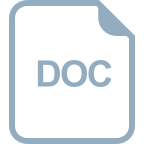
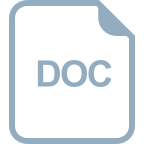
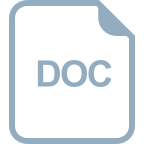