react 获取input的值
时间: 2023-08-11 13:08:43 浏览: 64
在 React 中获取 input 元素的值,可以通过两种方式:
1. 使用受控组件
受控组件是指表单元素的值被 React 组件的 state 控制。在 input 元素中添加 value 属性,并在 onChange 事件中更新 state 中的值即可获取 input 的值。
示例代码:
```
class InputValue extends React.Component {
constructor(props) {
super(props);
this.state = { value: '' };
this.handleChange = this.handleChange.bind(this);
}
handleChange(event) {
this.setState({ value: event.target.value });
}
render() {
return (
<div>
<input type="text" value={this.state.value} onChange={this.handleChange} />
<p>{this.state.value}</p>
</div>
);
}
}
```
2. 使用非受控组件
非受控组件是指表单元素的值不受 React 组件的 state 控制。在 input 元素中添加 ref 属性,并在提交表单时获取 input 元素的值即可获取 input 的值。
示例代码:
```
class InputValue extends React.Component {
constructor(props) {
super(props);
this.inputRef = React.createRef();
this.handleSubmit = this.handleSubmit.bind(this);
}
handleSubmit(event) {
event.preventDefault();
console.log(this.inputRef.current.value);
}
render() {
return (
<form onSubmit={this.handleSubmit}>
<input type="text" ref={this.inputRef} />
<button type="submit">Submit</button>
</form>
);
}
}
```
相关推荐
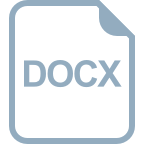
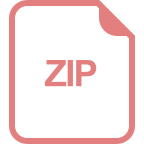
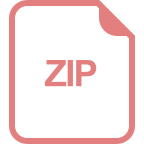
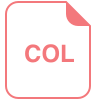
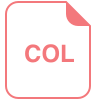
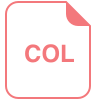
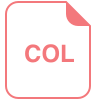
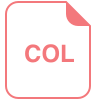









