头歌实践Python字符串之密码安全强度检查
时间: 2024-12-13 15:24:22 浏览: 6
头韵诗实践中,在Python中检测密码的安全强度,可以考虑以下几个方面:
1. **长度**:密码应该足够长,通常推荐至少12个字符。你可以编写函数检查密码长度是否超过指定阈值。
```python
def check_password_length(password):
MIN_LENGTH = 12
if len(password) < MIN_LENGTH:
return "密码太短,需要至少{}个字符".format(MIN_LENGTH)
else:
return "密码长度合适"
# 示例
print(check_password_length("strongPassword123")) # 输出: "密码长度合适"
```
2. **复杂性**:包括大写字母、小写字母、数字和特殊字符的组合。可以使用正则表达式来验证。
```python
import re
def check_complexity(password):
pattern = r"^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$"
if re.match(pattern, password):
return "密码包含复杂元素,很安全"
else:
return "密码需包含大小写字母、数字及特殊字符"
# 示例
print(check_complexity("S3cureP@ssw0rd")) # 输出: "密码包含复杂元素,很安全"
```
3. **避免常见词汇和生日等个人信息**:可以创建一个黑名单列表,检查密码是否包含其中的词。
```python
blacklist = ["password", "123456", "admin"]
def check_common_words(password):
for word in blacklist:
if word in password.lower():
return "密码不应包含常用词汇"
return "密码安全"
# 示例
print(check_common_words("Admin1234")) # 输出: "密码不应包含常用词汇"
```
阅读全文
相关推荐
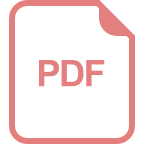
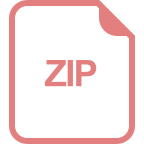
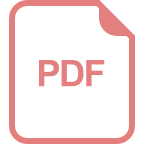















