springboot 复杂导入excel
时间: 2023-11-09 09:03:30 浏览: 49
可以使用 Apache POI 库来实现 Spring Boot 中的复杂导入 Excel。具体步骤如下:
1. 引入 Apache POI 依赖:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 创建 Excel 文件读取器:
```java
public class ExcelReader {
public static List<Map<String, Object>> readExcel(InputStream inputStream, String fileName) throws IOException {
Workbook workbook = null;
if (fileName.endsWith(".xls")) {
workbook = new HSSFWorkbook(inputStream);
} else if (fileName.endsWith(".xlsx")) {
workbook = new XSSFWorkbook(inputStream); }
Sheet sheet = workbook.getSheetAt(0);
List<Map<String, Object>> list = new ArrayList<>();
List<String> titles = new ArrayList<>();
for (int i = 0; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
if (row == null) {
continue;
}
Map<String, Object> map = new HashMap<>();
for (int j = 0; j < row.getLastCellNum(); j++) {
Cell cell = row.getCell(j);
if (cell == null) {
continue;
}
if (i == 0) {
titles.add(cell.getStringCellValue());
} else {
map.put(titles.get(j), getCellValue(cell));
}
}
if (i != 0) {
list.add(map);
}
}
return list;
}
private static Object getCellValue(Cell cell) {
Object value = null;
switch (cell.getCellType()) {
case STRING:
value = cell.getStringCellValue();
break;
case NUMERIC:
if (DateUtil.isCellDateFormatted(cell)) {
value = cell.getDateCellValue();
} else {
value = cell.getNumericCellValue();
}
break;
case BOOLEAN:
value = cell.getBooleanCellValue();
break;
case FORMULA:
value = cell.getCellFormula();
break;
default:
break;
}
return value;
}
}
```
3. 在 Controller 中使用 ExcelReader 读取 Excel 文件:
```java
@PostMapping("/import")
public String importExcel(@RequestParam("file") MultipartFile file) throws IOException {
List<Map<String, Object>> list = ExcelReader.readExcel(file.getInputStream(), file.getOriginalFilename());
// 处理 Excel 数据
return "success";
}
```
相关推荐
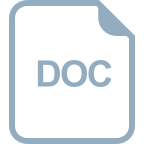
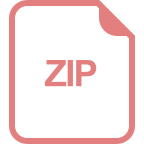














