dataframe将多个字符替换
时间: 2023-12-03 17:38:39 浏览: 19
以下是使用pandas.DataFrame.replace()方法将多个字符替换的示例代码:
```python
import pandas as pd
# 创建示例DataFrame
df = pd.DataFrame({'A': ['foo', 'bar', 'baz'], 'B': ['qux', 'quux', 'corge']})
# 将'A'列中的'foo'和'bar'替换为'new_value'
df['A'].replace(['foo', 'bar'], 'new_value', inplace=True)
# 将'B'列中的'qux'替换为'new_value1','quux'替换为'new_value2','corge'替换为'new_value3'
df['B'].replace({'qux': 'new_value1', 'quux': 'new_value2', 'corge': 'new_value3'}, inplace=True)
# 输出替换后的DataFrame
print(df)
```
输出结果为:
```
A B
0 new_value new_value1
1 new_value new_value2
2 baz new_value3
```
相关问题
dataframe 字符串替换
好的,针对您的问题,您可以使用 pandas 库中的 `replace` 方法来进行字符串替换。具体操作如下:
假设您有一个名为 `df` 的 DataFrame,其中有一列名为 `text`,您想要将其中的所有 "apple" 替换为 "orange",可以使用以下代码:
```python
df['text'] = df['text'].replace('apple', 'orange', regex=True)
```
其中,`regex=True` 表示使用正则表达式进行匹配替换。
如果您想要同时替换多个字符串,可以使用字典来指定替换规则,例如:
```python
replace_dict = {'apple': 'orange', 'banana': 'pear'}
df['text'] = df['text'].replace(replace_dict, regex=True)
```
这样就可以将所有 "apple" 替换为 "orange",将所有 "banana" 替换为 "pear"。
dataframe如何把列中指定字符串替换
可以使用pandas的replace方法来替换数据框中的指定字符串。
例如,假设我们有如下数据框:
```
import pandas as pd
df = pd.DataFrame({'A': ['apple', 'banana', 'cherry', 'apple'],
'B': [1, 2, 3, 4]})
print(df)
```
输出:
```
A B
0 apple 1
1 banana 2
2 cherry 3
3 apple 4
```
我们想把'A'列中的'apple'替换为'orange',可以使用replace方法:
```
df['A'] = df['A'].replace('apple', 'orange')
print(df)
```
输出:
```
A B
0 orange 1
1 banana 2
2 cherry 3
3 orange 4
```
如果想要替换多个字符串,可以将它们作为列表传递给replace方法:
```
df['A'] = df['A'].replace(['apple', 'banana'], 'fruit')
print(df)
```
输出:
```
A B
0 fruit 1
1 fruit 2
2 cherry 3
3 fruit 4
```
相关推荐
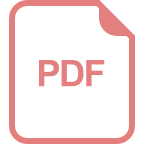
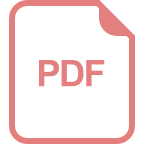
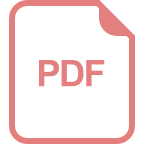












