解释代码:def get_images(dir_path): images_list = [] for img_path in glob.glob(dir_path + "*.jpg"): images_list.append(img_path) return images_list
时间: 2024-04-20 20:24:03 浏览: 67
这段代码定义了一个名为`get_images`的函数,该函数接受一个参数`dir_path`,代表一个目录的路径。函数的目的是在指定目录下查找所有以".jpg"结尾的文件,并将它们的路径存储在一个列表中。
代码中使用了`glob.glob`函数来获取指定目录下所有的文件路径,`glob.glob`函数使用通配符来匹配文件名。`dir_path + "*.jpg"`表示在`dir_path`目录下查找所有以".jpg"结尾的文件。
接下来,通过一个循环遍历获取到的文件路径,并将它们逐一添加到 `images_list` 列表中。
最后,函数返回存储了所有图片路径的列表 `images_list`。
相关问题
下面一段代码有什么错误:def evaluate_one_image(): workbook = xlsxwriter.Workbook('formatting.xlsx') worksheet = workbook.add_worksheet('My Worksheet') with tf.Graph().as_default(): BATCH_SIZE = 1 N_CLASSES = 4 image = tf.cast(image_array, tf.float32) image = tf.image.per_image_standardization(image) image = tf.reshape(image, [1, 208, 208, 3]) logit = model.cnn_inference(image, BATCH_SIZE, N_CLASSES) logit = tf.nn.softmax(logit) x = tf.placeholder(tf.float32, shape=[208, 208, 3]) logs_train_dir = 'log/' saver = tf.train.Saver() with tf.Session() as sess: print("从指定路径中加载模型...") ckpt = tf.train.get_checkpoint_state(logs_train_dir) if ckpt and ckpt.model_checkpoint_path: global_step = ckpt.model_checkpoint_path.split('/')[-1].split('-')[-1] saver.restore(sess, ckpt.model_checkpoint_path) print('模型加载成功, 训练的步数为: %s' % global_step) else: print('模型加载失败,checkpoint文件没找到!') prediction = sess.run(logit, feed_dict={x: image_array}) max_index = np.argmax(prediction) workbook.close() def evaluate_images(test_img): coord = tf.train.Coordinator() threads = tf.train.start_queue_runners(coord=coord) for index,img in enumerate(test_img): image = Image.open(img) image = image.resize([208, 208]) image_array = np.array(image) tf.compat.v1.threading.Thread(target=evaluate_one_image, args=(image_array, index)).start() # 请求停止所有线程 coord.request_stop() # 等待所有线程完成 coord.join(threads) if __name__ == '__main__': # 调用方法,开始测试 test_dir = 'data/test/' import glob import xlwt test_img = glob.glob(test_dir + '*.jpg') evaluate_images(test_img)
这段代码存在以下几个错误:
1. 缺少必要的库导入语句,例如`import tensorflow as tf`,`import numpy as np`,`import xlsxwriter`,`from PIL import Image`等。
2. 在`evaluate_one_image()`函数中,缺少了`image_array`参数的定义。
3. 缺少了`model`对象的定义和初始化。
4. 在`evaluate_images()`函数中,缺少了`image_array`参数的传递。
5. 在`evaluate_images()`函数中,缺少了线程的关闭操作。
以上是我对代码的分析,希望对你有所帮助!
def test(checkpoint_dir, style_name, test_dir, if_adjust_brightness, img_size=[256,256]): # tf.reset_default_graph() result_dir = 'results/'+style_name check_folder(result_dir) test_files = glob('{}/*.*'.format(test_dir)) test_real = tf.placeholder(tf.float32, [1, None, None, 3], name='test') with tf.variable_scope("generator", reuse=False): test_generated = generator.G_net(test_real).fake saver = tf.train.Saver() gpu_options = tf.GPUOptions(allow_growth=True) with tf.Session(config=tf.ConfigProto(allow_soft_placement=True, gpu_options=gpu_options)) as sess: # tf.global_variables_initializer().run() # load model ckpt = tf.train.get_checkpoint_state(checkpoint_dir) # checkpoint file information if ckpt and ckpt.model_checkpoint_path: ckpt_name = os.path.basename(ckpt.model_checkpoint_path) # first line saver.restore(sess, os.path.join(checkpoint_dir, ckpt_name)) print(" [*] Success to read {}".format(os.path.join(checkpoint_dir, ckpt_name))) else: print(" [*] Failed to find a checkpoint") return # stats_graph(tf.get_default_graph()) begin = time.time() for sample_file in tqdm(test_files) : # print('Processing image: ' + sample_file) sample_image = np.asarray(load_test_data(sample_file, img_size)) image_path = os.path.join(result_dir,'{0}'.format(os.path.basename(sample_file))) fake_img = sess.run(test_generated, feed_dict = {test_real : sample_image}) if if_adjust_brightness: save_images(fake_img, image_path, sample_file) else: save_images(fake_img, image_path, None) end = time.time() print(f'test-time: {end-begin} s') print(f'one image test time : {(end-begin)/len(test_files)} s'什么意思
这是一个 Python 函数,该函数接受五个参数:`checkpoint_dir`,`style_name`,`test_dir`,`if_adjust_brightness` 和 `img_size`。该函数使用 TensorFlow 实现了一个测试函数,用于测试训练好的 AnimeGAN V2 模型生成的效果。在函数中,首先使用 `glob` 函数获取测试图片的路径列表,然后使用 TensorFlow 定义了一个占位符 `test_real`,用于输入测试图片。接着,使用 AnimeGAN V2 模型生成器 `generator.G_net` 生成虚假图像 `test_generated`。随后,使用 TensorFlow 定义了一个 Saver 对象,用于从检查点文件中恢复模型。最后,使用 TensorFlow Session 对象运行生成器模型,生成对应的虚假图像,并将其保存到指定的目录中。
其中,如果 `if_adjust_brightness` 为真,则调用 `save_images` 函数保存虚假图像,并调用 `load_test_data` 函数加载测试图片并调整亮度;否则,调用 `save_images` 函数保存虚假图像,但不会调用 `load_test_data` 函数调整亮度。函数还输出了测试的总时间和每张图片测试所需的时间。
阅读全文
相关推荐
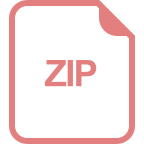
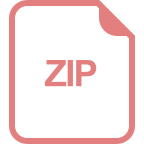
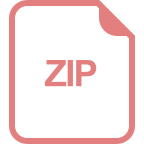












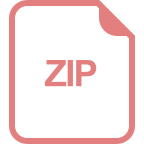
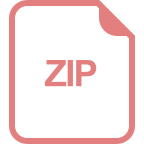