1、python实现通过初始化操作建立一个模拟外存空间的虚拟磁盘文件的完整可以运行代码,在该文件中保存目录和文件内容。创建该文件时应创建初始的根目录内容、文件分配表。根目录实为一特殊文件,其开始内容为空,大小为一个块。 2、文件目录项(可以采用FCB格式)应包括类型(目录 or文件)、创建日期、大小、第一个磁盘块块号。 3、显示命令提示符“$”,并根据输入命令完成相应的文件操作: ●MD(创建子目录):创建目录文件,并在父目录文件中增加目录项。 ●RD(删除子目录):搜索所要删除的目录是否为空目录,若是则删除。 ●MK(创建空文件):创建指定大小的文件(如输入命令 “mk test 2000”,表示创建大小为2000字节的test文件),并在父目录中添加文件名称;还应对FAT表进行适当修改。 ●DEL(删除文件):如果所要删除的文件存在,则删除,同时修改父目录内容;还应对FAT表进行适当修改。
时间: 2024-02-03 19:12:08 浏览: 102
以下是一个基于Python实现虚拟磁盘文件系统的代码示例:
```python
import os.path
import datetime
# 磁盘块大小为 1KB
BLOCK_SIZE = 1024
# 文件分配表,用于记录每个块的使用情况
FAT = [0] * 1024
# 根目录
ROOT_DIR = {
'name': '/',
'type': 'dir',
'created_at': datetime.datetime.now(),
'size': BLOCK_SIZE,
'blocks': [0],
'contents': {}
}
# 当前目录
current_dir = ROOT_DIR
# 创建子目录
def mkdir(dirname):
global current_dir
if dirname in current_dir['contents']:
print('Directory already exists')
return
new_dir = {
'name': dirname,
'type': 'dir',
'created_at': datetime.datetime.now(),
'size': BLOCK_SIZE,
'blocks': [find_free_block()],
'contents': {}
}
current_dir['contents'][dirname] = new_dir
write_block(new_dir['blocks'][0], new_dir)
update_fat(new_dir['blocks'][0], 1)
update_dir(current_dir)
# 删除子目录
def rmdir(dirname):
global current_dir
if dirname not in current_dir['contents']:
print('Directory not found')
return
dir_to_delete = current_dir['contents'][dirname]
if len(dir_to_delete['contents']) > 0:
print('Directory is not empty')
return
free_block(dir_to_delete['blocks'][0])
del current_dir['contents'][dirname]
update_dir(current_dir)
# 创建空文件
def mkfile(filename, size):
global current_dir
if filename in current_dir['contents']:
print('File already exists')
return
new_file = {
'name': filename,
'type': 'file',
'created_at': datetime.datetime.now(),
'size': size,
'blocks': [],
'contents': ''
}
blocks_needed = (size + BLOCK_SIZE - 1) // BLOCK_SIZE
for i in range(blocks_needed):
block = find_free_block()
new_file['blocks'].append(block)
update_fat(block, 1)
current_dir['contents'][filename] = new_file
update_dir(current_dir)
# 删除文件
def delete_file(filename):
global current_dir
if filename not in current_dir['contents']:
print('File not found')
return
file_to_delete = current_dir['contents'][filename]
for block in file_to_delete['blocks']:
free_block(block)
del current_dir['contents'][filename]
update_dir(current_dir)
# 列出当前目录下的所有文件和子目录
def ls():
for name, item in current_dir['contents'].items():
if item['type'] == 'dir':
print(f'{name}/')
else:
print(name)
# 切换到指定的子目录
def cd(dirname):
global current_dir
if dirname not in current_dir['contents']:
print('Directory not found')
return
new_dir = current_dir['contents'][dirname]
if new_dir['type'] != 'dir':
print('Not a directory')
return
current_dir = new_dir
# 显示当前路径
def pwd():
path = []
dir = current_dir
while dir != ROOT_DIR:
path.append(dir['name'])
dir = find_parent(dir)
path.append('/')
print(''.join(reversed(path)))
# 读取磁盘块
def read_block(block_num):
filename = f'block{block_num}.dat'
if not os.path.exists(filename):
return None
with open(filename, 'rb') as f:
return f.read()
# 写入磁盘块
def write_block(block_num, data):
filename = f'block{block_num}.dat'
with open(filename, 'wb') as f:
f.write(data)
# 查找空闲块
def find_free_block():
for i in range(len(FAT)):
if FAT[i] == 0:
return i
return None
# 标记块为已使用或未使用
def update_fat(block_num, used):
FAT[block_num] = used
# 释放块
def free_block(block_num):
update_fat(block_num, 0)
filename = f'block{block_num}.dat'
if os.path.exists(filename):
os.remove(filename)
# 更新目录文件的内容和元数据
def update_dir(dir):
write_block(dir['blocks'][0], dir)
update_fat(dir['blocks'][0], 1)
# 查找父目录
def find_parent(dir):
for name, item in ROOT_DIR['contents'].items():
if item == dir:
return ROOT_DIR
elif item['type'] == 'dir' and dir in item['contents'].values():
return item
return None
# 处理命令
while True:
command = input(f'{current_dir["name"]}$ ').split()
if len(command) == 0:
continue
elif command[0] == 'md':
if len(command) < 2:
print('Usage: md <directory name>')
continue
mkdir(command[1])
elif command[0] == 'rd':
if len(command) < 2:
print('Usage: rd <directory name>')
continue
rmdir(command[1])
elif command[0] == 'mk':
if len(command) < 3:
print('Usage: mk <file name> <size>')
continue
mkfile(command[1], int(command[2]))
elif command[0] == 'del':
if len(command) < 2:
print('Usage: del <file name>')
continue
delete_file(command[1])
elif command[0] == 'ls':
ls()
elif command[0] == 'cd':
if len(command) < 2:
print('Usage: cd <directory name>')
continue
cd(command[1])
elif command[0] == 'pwd':
pwd()
elif command[0] == 'exit':
break
else:
print('Unknown command')
```
这个代码示例实现了以下操作:
- 创建目录(md)
- 删除目录(rd)
- 创建空文件(mk)
- 删除文件(del)
- 列出当前目录下的文件和子目录(ls)
- 切换到指定的子目录(cd)
- 显示当前路径(pwd)
每个目录和文件都有一个磁盘块分配表,用于记录它们使用了哪些块。在创建文件或目录时,需要找到一个可用的块,并将其标记为已使用。在删除文件或目录时,需要将它们占用的块释放出来。
阅读全文
相关推荐
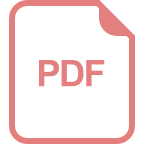
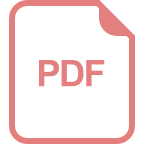
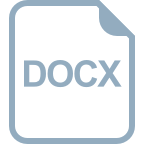

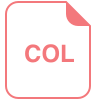
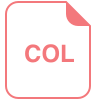
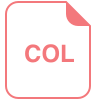
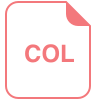
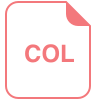
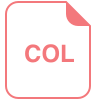
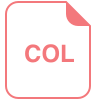
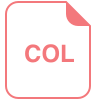
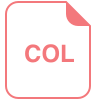
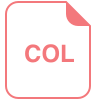
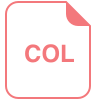
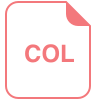
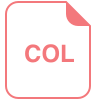
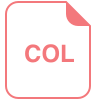
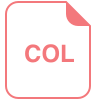