c语言中用read函数读取图片像素数据
时间: 2024-05-03 19:19:20 浏览: 96
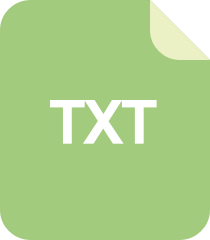
基于C语言图片数据读出
在C语言中,可以使用read函数从文件中读取二进制数据,包括图片像素数据。下面是一个读取BMP格式图片像素数据的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
typedef unsigned char byte;
typedef unsigned short word;
typedef unsigned int dword;
#pragma pack(push, 1)
// BMP文件头结构体
typedef struct {
word bfType; // 文件类型
dword bfSize; // 文件大小
word bfReserved1; // 保留字段
word bfReserved2; // 保留字段
dword bfOffBits; // 数据偏移量
} BMPFileHeader;
// BMP信息头结构体
typedef struct {
dword biSize; // 结构体大小
dword biWidth; // 图像宽度
dword biHeight; // 图像高度
word biPlanes; // 颜色平面数
word biBitCount; // 每个像素的位数
dword biCompression; // 压缩类型
dword biSizeImage; // 图像数据大小
dword biXPelsPerMeter; // 水平分辨率
dword biYPelsPerMeter; // 垂直分辨率
dword biClrUsed; // 使用的颜色数
dword biClrImportant; // 重要的颜色数
} BMPInfoHeader;
#pragma pack(pop)
int main() {
BMPFileHeader fh;
BMPInfoHeader ih;
byte* data;
int fd = open("test.bmp", O_RDONLY);
if (fd == -1) {
perror("open");
return -1;
}
// 读取文件头和信息头
read(fd, &fh, sizeof(fh));
read(fd, &ih, sizeof(ih));
// 分配像素数据内存
data = (byte*)malloc(ih.biSizeImage);
if (data == NULL) {
perror("malloc");
return -1;
}
// 读取像素数据
lseek(fd, fh.bfOffBits, SEEK_SET);
read(fd, data, ih.biSizeImage);
close(fd);
// 处理像素数据,这里只是简单地打印第一个像素的RGB值
byte* pixel = data;
printf("R:%d G:%d B:%d\n", pixel[2], pixel[1], pixel[0]);
// 释放内存
free(data);
return 0;
}
```
需要注意的是,不同图片格式的像素数据存储方式不同,因此读取方式也会有所差异。此处只是针对BMP格式进行了简单的示例,其他格式需要根据具体情况进行处理。
阅读全文
相关推荐
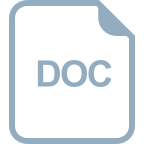
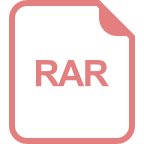
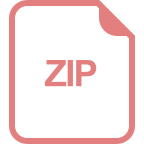
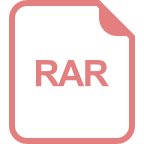
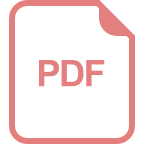
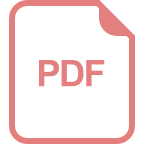
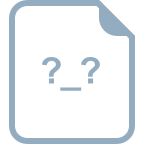
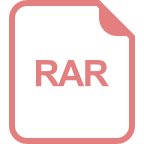
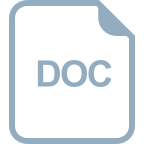
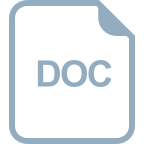




