当我给python解压gzip内容的多种代码,给我代码
时间: 2023-09-26 10:06:27 浏览: 85
### 回答1:
答:你可以试试下面的代码:import gzipwith gzip.open('file.txt.gz', 'rb') as f:
file_content = f.read()print(file_content)
### 回答2:
当使用Python解压gzip内容时,可以使用以下多种代码实现。
1. 使用gzip模块进行解压:
```python
import gzip
def unzip_gzip(file_path, output_path):
with gzip.open(file_path, 'rb') as f:
with open(output_path, 'wb') as output_file:
output_file.write(f.read())
# 使用示例
file_path = 'compressed_file.gz'
output_path = 'uncompressed_file.txt'
unzip_gzip(file_path, output_path)
```
2. 使用shutil模块中的copyfileobj方法:
```python
import gzip
import shutil
def unzip_gzip(file_path, output_path):
with open(file_path, 'rb') as f_in:
with open(output_path, 'wb') as f_out:
with gzip.GzipFile(fileobj=f_in) as uncompressed:
shutil.copyfileobj(uncompressed, f_out)
# 使用示例
file_path = 'compressed_file.gz'
output_path = 'uncompressed_file.txt'
unzip_gzip(file_path, output_path)
```
3. 使用zlib模块进行解压:
```python
import zlib
def unzip_gzip(file_path, output_path):
with open(file_path, 'rb') as f:
compressed_data = f.read()
uncompressed_data = zlib.decompress(compressed_data, zlib.MAX_WBITS | 16)
with open(output_path, 'wb') as output_file:
output_file.write(uncompressed_data)
# 使用示例
file_path = 'compressed_file.gz'
output_path = 'uncompressed_file.txt'
unzip_gzip(file_path, output_path)
```
以上代码中,file_path是压缩文件的路径,output_path是解压后文件的输出路径。通过以上代码,可以将gzip压缩文件解压成普通的文本文件,从而对其进行处理。
### 回答3:
以下是三种用Python解压gzip内容的方法:
方法一:
```python
import gzip
def decompress_gzip(file_path):
with gzip.open(file_path, 'rb') as f:
decompressed_data = f.read().decode('utf-8')
return decompressed_data
```
使用gzip模块的open函数打开gzip压缩文件,以二进制模式('rb')读取文件内容,并使用decode函数将解压后的数据转换成utf-8编码格式。
方法二:
```python
import gzip
import shutil
def decompress_gzip(file_path):
with gzip.open(file_path, 'rb') as f_in:
with open('output.txt', 'wb') as f_out:
shutil.copyfileobj(f_in, f_out)
```
使用gzip模块的open函数打开gzip压缩文件,并以二进制模式('rb')读取文件内容。然后使用shutil模块的copyfileobj函数将解压后的数据写入到新文件output.txt中。
方法三:
```python
import gzip
def decompress_gzip(file_path):
with open(file_path, 'rb') as f:
gzip_file = gzip.GzipFile(fileobj=f)
decompressed_data = gzip_file.read().decode('utf-8')
return decompressed_data
```
使用gzip模块的GzipFile函数打开gzip压缩文件,并将其传递给gzip.GzipFile对象。然后读取解压后的数据并使用decode函数将其转换成utf-8编码格式。
阅读全文
相关推荐
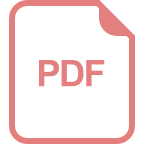
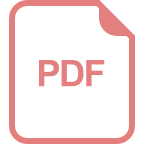

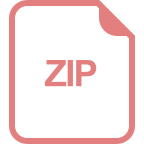
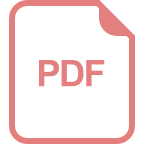
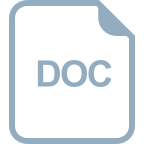
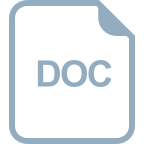
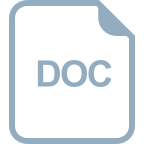
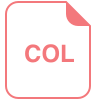
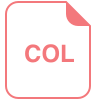
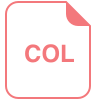
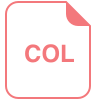
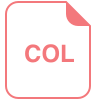
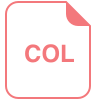
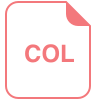
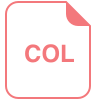
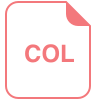
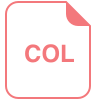