你是否有实现实现成绩查询的C语言代码
时间: 2024-09-13 18:16:19 浏览: 36
在C语言中实现实现成绩查询功能,通常会涉及到结构体的使用,数组的操作,以及对成绩的排序和查找算法。下面是一个简单的示例,通过结构体来保存学生的学号和成绩,并使用一个函数来实现查询功能。
首先定义一个学生结构体和成绩信息:
```c
#include <stdio.h>
#include <string.h>
// 定义学生信息结构体
typedef struct {
char student_id[20]; // 学号
char name[50]; // 姓名
float score; // 成绩
} Student;
// 函数声明
int find_student_index(Student students[], int length, const char* student_id);
void print_student_info(Student student);
int main() {
// 假设有一个学生信息数组
Student students[] = {
{"2019001", "张三", 86.5},
{"2019002", "李四", 92.0},
{"2019003", "王五", 78.0}
};
int length = sizeof(students) / sizeof(students[0]);
char target_id[20];
// 查询学生信息
printf("请输入要查询的学生学号: ");
scanf("%s", target_id);
int index = find_student_index(students, length, target_id);
if (index != -1) {
print_student_info(students[index]);
} else {
printf("未找到学号为 %s 的学生。\n", target_id);
}
return 0;
}
// 根据学号查找学生在数组中的索引,未找到返回-1
int find_student_index(Student students[], int length, const char* student_id) {
for (int i = 0; i < length; i++) {
if (strcmp(students[i].student_id, student_id) == 0) {
return i;
}
}
return -1;
}
// 打印学生信息
void print_student_info(Student student) {
printf("学号:%s\n", student.student_id);
printf("姓名:%s\n", student.name);
printf("成绩:%.2f\n", student.score);
}
```
在上述代码中,我们首先定义了一个`Student`结构体来保存每个学生的学号、姓名和成绩。然后在`main`函数中,创建了一个`Student`数组来模拟学生信息的集合,并通过`find_student_index`函数根据学号查找学生信息在数组中的索引。如果找到了,就通过`print_student_info`函数打印出学生的信息。如果未找到,则输出未找到学生的信息。
阅读全文
相关推荐
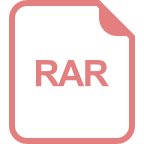
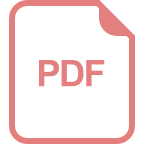
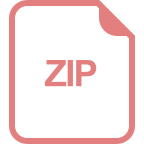
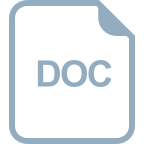
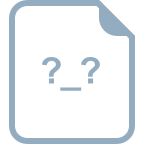
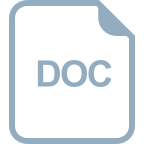
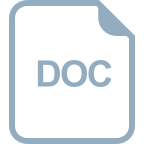
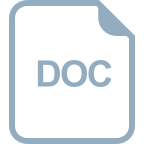
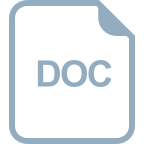
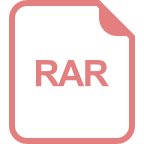
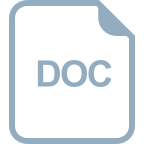

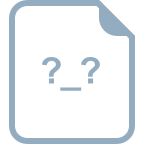
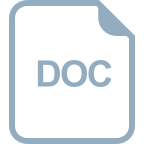
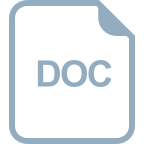
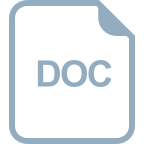
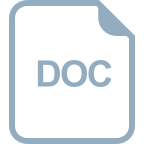