1请按照我们刚才所说的知识,现在班里面有个同学叫做坤坤,请通过健盘输入去登记他的名字,身高,体重,年龄,性别等信息,并且将其信息存放到一个结构体里面 2,将上面的坤坤的结构体信息存储到字符串数组当中,并打印出来sprintf 3,尝试将第2题中的字符串数组信息提取出来,放到另外一个结构体变量当中sscanf
时间: 2024-04-08 12:33:12 浏览: 21
1. 好的,请提供坤坤的姓名、身高、体重、年龄和性别信息。
坤坤的姓名:坤坤
身高(cm):170
体重(kg):60
年龄:18
性别:男
以下是将坤坤的信息存放到一个结构体中的示例代码:
```cpp
#include <stdio.h>
struct Student {
char name[20];
int height;
int weight;
int age;
char gender[10];
};
int main() {
struct Student kun;
printf("请输入坤坤的姓名:");
scanf("%s", kun.name);
printf("请输入坤坤的身高(cm):");
scanf("%d", &kun.height);
printf("请输入坤坤的体重(kg):");
scanf("%d", &kun.weight);
printf("请输入坤坤的年龄:");
scanf("%d", &kun.age);
printf("请输入坤坤的性别:");
scanf("%s", kun.gender);
// 打印坤坤的信息
printf("\n");
printf("姓名:%s\n", kun.name);
printf("身高:%dcm\n", kun.height);
printf("体重:%dkg\n", kun.weight);
printf("年龄:%d岁\n", kun.age);
printf("性别:%s\n", kun.gender);
return 0;
}
```
2. 现在将上面的坤坤的结构体信息存储到字符串数组中,并打印出来。请提供一个字符串数组的名称。
假设字符串数组的名称为 `studentInfo`,以下是将坤坤的结构体信息存储到字符串数组中,并使用 `sprintf` 打印出来的示例代码:
```cpp
#include <stdio.h>
struct Student {
char name[20];
int height;
int weight;
int age;
char gender[10];
};
int main() {
struct Student kun;
char studentInfo[100];
// 坤坤的信息赋值给结构体
sprintf(kun.name, "坤坤");
kun.height = 170;
kun.weight = 60;
kun.age = 18;
sprintf(kun.gender, "男");
// 将结构体信息存储到字符串数组
sprintf(studentInfo, "姓名:%s\n身高:%dcm\n体重:%dkg\n年龄:%d岁\n性别:%s\n", kun.name, kun.height, kun.weight, kun.age, kun.gender);
// 打印字符串数组中的信息
printf("%s\n", studentInfo);
return 0;
}
```
3. 现在尝试将第2题中的字符串数组信息提取出来,放到另外一个结构体变量中。请提供另外一个结构体变量的名称。
假设另外一个结构体变量的名称为 `extractedInfo`,以下是将字符串数组信息提取出来放到另外一个结构体变量中的示例代码:
```cpp
#include <stdio.h>
struct Student {
char name[20];
int height;
int weight;
int age;
char gender[10];
};
int main() {
struct Student extractedInfo;
char studentInfo[100] = "姓名:坤坤\n身高:170cm\n体重:60kg\n年龄:18岁\n性别:男\n";
// 从字符串数组中提取信息到结构体
sscanf(studentInfo, "姓名:%s\n身高:%dcm\n体重:%dkg\n年龄:%d岁\n性别:%s\n", extractedInfo.name, &extractedInfo.height, &extractedInfo.weight, &extractedInfo.age, extractedInfo.gender);
// 打印提取出的信息
printf("姓名:%s\n", extractedInfo.name);
printf("身高:%dcm\n", extractedInfo.height);
printf("体重:%dkg\n", extractedInfo.weight);
printf("年龄:%d岁\n", extractedInfo.age);
printf("性别:%s\n", extractedInfo.gender);
return 0;
}
```
请注意,在提取字符串数组信息时,使用 `sscanf` 函数并提供对应的格式化字符串,以确保正确提取每个数据项。
相关推荐
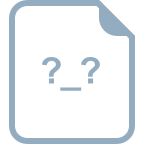
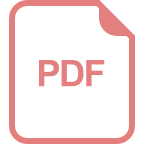
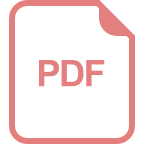













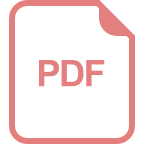
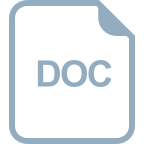