输入一个正整数n,找到n中最小的数位数字x,统计x出现的次数s,以及x最左侧的出现位置lpos,不用std::string
时间: 2024-12-20 07:19:58 浏览: 5
要解决这个问题,我们可以创建一个简单的函数来遍历输入的整数 `n`,找出其中最小的数字 `x`、它的出现次数 `s`,以及首次出现的位置 `lpos`。由于我们不使用 `std::string`,我们将直接处理整数。
```cpp
#include <iostream>
// 函数用于计算最小数字及其出现次数和位置
void findMinDigit(int n, int& x, int& s, int& lpos) {
// 初始化
x = n;
s = 0;
lpos = -1;
// 遍历每一位
while (n != 0) {
int digit = n % 10; // 获取个位数字
if (digit < x) { // 如果找到了更小的数字
x = digit; // 更新最小数字
s = 1; // 初始出现次数为1
lpos = n / 10; // 记录当前位置
} else if (digit == x) { // 如果找到相同的数字,计数加一
++s;
}
n /= 10; // 移除最低位
}
// 如果最小数字就是0,则默认从左起第一个非零位开始
if (x == 0 && lpos == -1) {
for (int i = 1; i <= n; ++i) {
if (n % 10 != 0) {
lpos = i;
break;
}
n /= 10;
}
}
}
int main() {
int n;
std::cout << "Enter a positive integer: ";
std::cin >> n;
int min_digit, count, leftmost_pos;
findMinDigit(n, min_digit, count, leftmost_pos);
std::cout << "The smallest digit is " << min_digit << ", it appears " << count << " times, and its first occurrence is at position " << leftmost_pos << std::endl;
return 0;
}
```
在这个代码中,我们定义了一个名为 `findMinDigit` 的函数,该函数接受整数 `n` 和三个引用参数(`x`, `s`, 和 `lpos`),分别代表最小的数字、出现次数和首次出现的位置。然后我们通过循环逐位处理整数,并更新这些变量。在 `main` 函数中,我们读取用户的输入并调用这个函数来获取结果。
阅读全文
相关推荐
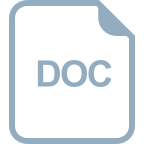
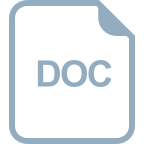
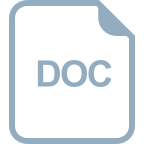





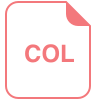
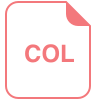
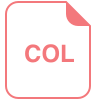
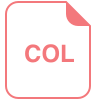
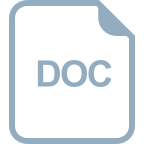
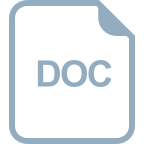
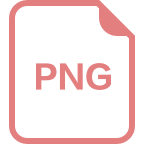
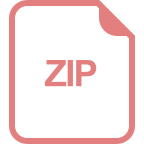