给出完整的,具体的C代码
时间: 2024-12-17 16:50:56 浏览: 3
当然,下面是关于C语言中栈应用的完整示例代码,包括一个简单的前缀到后缀表达式转换函数和一个后缀表达式的求值函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 栈结构体
typedef struct StackNode {
int data;
struct StackNode* next;
} StackNode;
// 创建新栈节点
StackNode* createStackNode(int data) {
StackNode* newNode = (StackNode*)malloc(sizeof(StackNode));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 判断字符串是否全为数字
int isNumber(const char* str) {
int i;
for (i = 0; str[i] != '\0'; i++) {
if (!isdigit(str[i])) {
return 0;
}
}
return 1;
}
// 入栈操作
void push(StackNode** top, int value) {
StackNode* newNode = createStackNode(value);
newNode->next = *top;
*top = newNode;
}
// 出栈操作
int pop(StackNode** top) {
if (*top == NULL) {
return -1;
}
StackNode* temp = *top;
int value = temp->data;
*top = temp->next;
free(temp);
return value;
}
// 计算两个数和的结果
int calculate(int a, int b, char op) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
if (b != 0)
return a / b;
else
return 0; // 处理除数为零的情况
default:
return -1; // 未知操作符
}
}
// 前缀到后缀表达式转换
void prefixToPostfix(char* infix) {
StackNode* top = NULL;
char* token = strtok(infix, "+-*/()"); // 分割成令牌
while (token != NULL) {
if (isNumber(token)) { // 数字直接压入栈
push(&top, atoi(token));
} else if (token[0] == '(') { // 左括号进栈
push(&top, token[0]);
} else if (token[0] == ')') { // 右括号出栈计算并进栈
while (top->data != '(') {
int result = calculate(pop(&top), pop(&top), token[0]);
if (result >= 0) {
push(&top, result);
}
}
push(&top, ')'); // 弹出剩余的左括号
} else { // 遇到操作符
while (top->data != '\0' && precedence(token[0]) <= precedence(top->data)) {
int result = calculate(pop(&top), pop(&top), top->data);
if (result >= 0) {
push(&top, result);
}
}
push(&top, token[0]); // 将操作符压入栈
}
token = strtok(NULL, "+-*/()");
}
// 最后一次出栈并打印结果
while (top->data != '\0') {
int result = calculate(pop(&top), pop(&top), top->data);
if (result >= 0) {
printf("%d ", result);
}
}
}
// 后缀表达式求值
int evaluatePostfix(char* postfix) {
StackNode* top = NULL;
for (char* token = postfix; *token != '\0'; token++) {
if (isNumber(token)) { // 数字入栈
push(&top, atoi(token));
} else { // 操作符出栈两个数并计算
int b = pop(&top);
int a = pop(&top);
int result = calculate(a, b, *token);
if (result >= 0) {
push(&top, result);
}
}
}
return pop(&top);
}
int main() {
char infix[] = "3+4*5";
char postfix[100];
prefixToPostfix(infix);
printf("Prefix to Postfix: ");
printf("%s\n", postfix);
int result = evaluatePostfix(postfix);
printf("Evaluation: %d\n", result);
return 0;
}
```
这个例子演示了如何将前缀表达式转换为后缀表达式,并计算后缀表达式的结果。注意,实际应用中可能需要更完善的错误处理和优化。
阅读全文
相关推荐
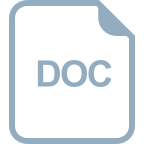
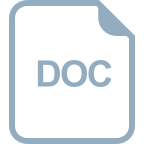
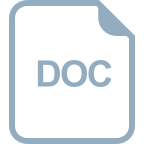
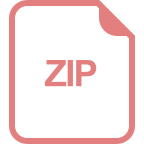
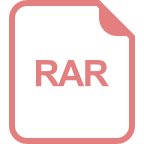
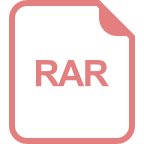
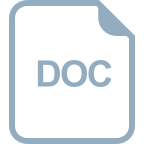
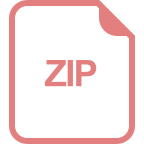
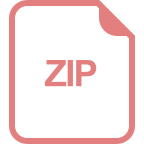
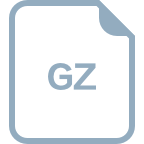
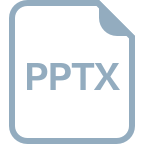
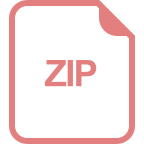
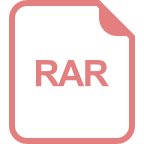
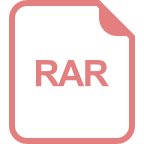
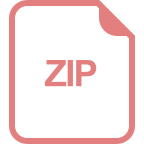
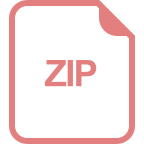
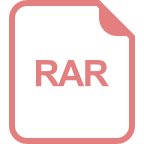
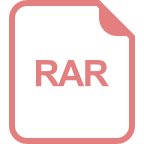