数据结构课程设计通讯录管理系统哈希表
时间: 2024-01-12 13:04:42 浏览: 109
通讯录管理系统可以使用哈希表来实现快速的电话号码查找。下面是一个简单的示例代码,演示了如何使用哈希表来实现通讯录管理系统:
```python
class Contact:
def __init__(self, phone_number, username, address):
self.phone_number = phone_number
self.username = username
self.address = address
class HashTable:
def __init__(self):
self.table = {}
def insert(self, contact):
key = contact.phone_number
if key not in self.table:
self.table[key] = contact
def search_by_phone_number(self, phone_number):
if phone_number in self.table:
return self.table[phone_number]
else:
return None
def search_by_username(self, username):
for contact in self.table.values():
if contact.username == username:
return contact
return None
# 创建哈希表
phone_number_table = HashTable()
username_table = HashTable()
# 从文件中读取记录并插入哈希表
with open('contacts.txt', 'r') as file:
for line in file:
phone_number, username, address = line.strip().split(',')
contact = Contact(phone_number, username, address)
phone_number_table.insert(contact)
username_table.insert(contact)
# 根据电话号码查找联系人
phone_number = '1234567890'
contact = phone_number_table.search_by_phone_number(phone_number)
if contact:
print(f"Contact found - Phone Number: {contact.phone_number}, Username: {contact.username}, Address: {contact.address}")
else:
print("Contact not found")
# 根据用户名查找联系人
username = 'John Doe'
contact = username_table.search_by_username(username)
if contact:
print(f"Contact found - Phone Number: {contact.phone_number}, Username: {contact.username}, Address: {contact.address}")
else:
print("Contact not found")
```
这个示例代码中,我们定义了一个Contact类来表示每个联系人的信息。然后,我们创建了两个哈希表,一个以电话号码为关键字,另一个以用户名为关键字。我们从文件中读取记录,并将每个记录插入到两个哈希表中。最后,我们可以根据电话号码或用户名来查找联系人。
阅读全文
相关推荐




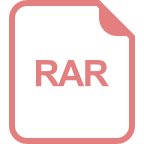
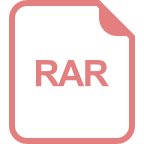


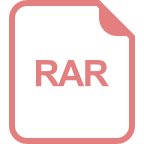

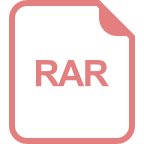







