使用【C++】写一个复杂的【成语接龙游戏】的代码,语言为中文
时间: 2024-12-20 14:19:16 浏览: 12
要编写一个复杂的成语接龙游戏,我们可以按照以下步骤设计程序:
1. 定义数据结构:存储成语及其相关的下一位成语。可以用关联数组(如`std::unordered_map`)来存储每个成语及其下一个成语。
2. 成语库:准备一份成语列表,可以包含多个成语,方便游戏中查询。
3. 游戏逻辑:初始化游戏,生成随机成语,玩家输入成语进行验证,如果正确则继续,否则提示错误并提供下一位成语的首字母帮助。
4. 循环游戏:直到游戏结束或达到特定轮数。
5. 结束条件:比如连续答对一定数量的成语后赢得游戏,或者无法接上时结束。
以下是一个简单的框架代码示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
#include <cctype>
// 定义成语及其下一个成语的数据结构
struct Chengyu {
std::string id; // 成语ID
std::string word; // 成语字串
char next_char; // 下一个成语的首字母
};
class Game {
private:
std::vector<Chengyu> chengyu_list;
int current_chengyu_index;
public:
Game(std::vector<std::string>& chengyu_data) {
// 填充成语库
for (const auto& word : chengyu_data) {
Chengyu c;
c.id = word;
c.word = tolower(word); // 将成语转换为小写便于比较
c.next_char = word.back(); // 获取下一个成语的首字母
chengyu_list.push_back(c);
}
// 随机选择初始成语
current_chengyu_index = rand() % chengyu_list.size();
}
bool play_round() {
std::cout << "请输入下一个成语:" << chengyu_list[current_chengyu_index].word << "的下一个成语:";
std::string user_input;
std::cin >> user_input;
// 检查用户输入是否匹配
if (tolower(user_input) == chengyu_list[current_chengyu_index].word.substr(1)) {
current_chengyu_index++;
if (current_chengyu_index == chengyu_list.size()) {
return false; // 如果到达末尾,游戏结束
}
std::cout << "正确!下一个成语是:" << chengyu_list[current_chengyu_index].word << ",首字母是" << chengyu_list[current_chengyu_index].next_char << std::endl;
} else {
std::cout << "错误,请重新输入:" << chengyu_list[current_chengyu_index].next_char << std::endl;
}
return true;
}
void start_game(int max_rounds) {
for (int i = 0; i < max_rounds && play_round(); ++i) {
std::cout << "第" << (i + 1) << "轮:" << std::endl;
}
std::cout << "游戏结束!" << std::endl;
}
};
int main() {
// 示例成语库
std::vector<std::string> chengyu_data = {"接二连三", "三心二意", "意气风发", "发扬光大", ...}; // 这里填写完整成语列表
Game game(chengyu_data);
game.start_game(10); // 设置最多进行10轮游戏
return 0;
}
```
请注意,这只是一个基础版本,实际游戏中可能还需要添加错误处理、用户界面优化以及更多的游戏规则功能。此外,你需要自己准备一个完整的成语列表,并确保成语库中的成语按照某种顺序排列以便形成循环接龙。你可以根据需要扩展这个框架。如果你需要更详细的实现或者有其他具体需求,请告诉我。
阅读全文
相关推荐
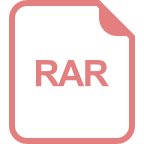
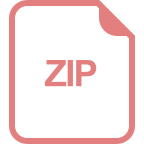
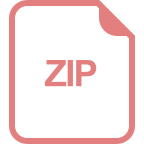
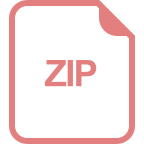
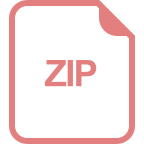
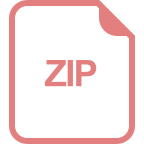
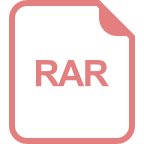
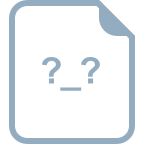
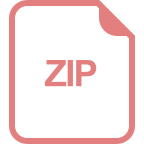
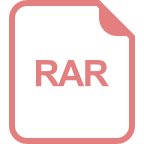
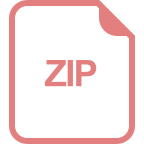
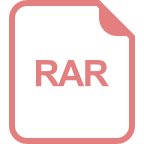
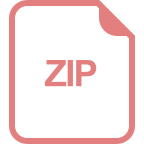