用最新的Python写一个程序,将指定的文字插入到指定的PDF文件指定位置
时间: 2024-10-25 21:08:42 浏览: 48
在Python中,我们可以使用`PyPDF2`库来读取PDF文件,并通过`pdfplumber`库来更方便地操作文本和页面。以下是一个简单的示例程序,它演示了如何打开PDF文件、定位插入点并添加新的文字:
```python
from PyPDF2 import PdfFileWriter, PdfFileReader
import pdfplumber
# 定义要插入的文本和目标PDF文件路径
text_to_insert = "这是一段插入的内容"
pdf_path = 'example.pdf'
# 使用pdfplumber打开PDF
with pdfplumber.open(pdf_path) as pdf:
# 获取第一页
page = pdf.pages[0]
# 获取当前页的宽度和高度
width, height = page.width, page.height
# 创建一个新的PdfFileWriter实例用于写入操作
writer = PdfFileWriter()
# 读取原PDF
reader = PdfFileReader(pdf_path)
for i in range(reader.getNumPages()):
# 将每一页复制到writer
writer.addPage(reader.getPage(i))
# 找到插入位置,这里假设我们想在页面底部中央插入
insert_y = height - 50 # 插入位置Y坐标,你可以根据需要调整
insert_x = width / 2 # 插入位置X坐标,同样可以自定义
# 创建一个矩形框表示插入区域
box = (insert_x - len(text_to_insert) * 10, insert_y, insert_x, insert_y + 20)
# 写入新的文本到PDF
text_obj = writer.add_annot('Text', rectangle=box)
text_obj.update({"/Contents": bytes(text_to_insert.encode('utf-8'))})
# 将修改后的PDF保存
output_path = 'modified_example.pdf'
with open(output_path, 'wb') as out_file:
writer.write(out_file)
print(f"文本已成功插入到PDF的{insert_x}, {insert_y}坐标。新PDF保存为{output_path}")
阅读全文
相关推荐
















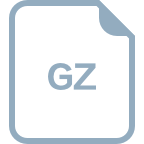

