分别统计字符串中大写字母和小写字母的个数。例如,输入字符串s:“AAaaBBb123CCccccd”,则应输出结果:upper=6,lower=3。 C语言
时间: 2024-12-23 12:20:55 浏览: 8
在C语言中,你可以使用`ctype.h`库提供的函数来分别统计字符串中的大写字母和小写字母。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
int main() {
char s[] = "AAaaBBb123CCccccd";
int upper = 0, lower = 0;
for (int i = 0; s[i] != '\0'; i++) { // 遍历整个字符串
if (isupper(s[i])) { // 检查字符是否为大写字母
upper++;
} else if (islower(s[i])) { // 检查字符是否为小写字母
lower++;
}
}
printf("upper=%d, lower=%d\n", upper, lower); // 输出结果
return 0;
}
```
这个程序首先初始化两个计数器(upper和lower),然后遍历字符串`s`,使用`isupper()`检查字符是否为大写字母,如果是就增加`upper`;用`islower()`检查是否为小写字母,如果是就增加`lower`。最后,打印出大写字母和小写字母的数量。
相关问题
分别统计字符串中大写字母和小写字母的个数。例如,输入字符串s:“AAaaBBb123CCccccd”,则应输出结果:upper=6,lower=3。
在编程中,统计字符串中大写字母和小写字母的数量通常是一个简单的文本处理任务。你可以通过遍历字符串并检查每个字符的ASCII值来实现这个功能。在Python中,可以这样做:
```python
def count_letters(s):
upper = sum(1 for c in s if c.isupper()) # 使用isupper()检查大写字母
lower = sum(1 for c in s if c.islower()) # 使用islower()检查小写字母
return "upper={}, lower={}".format(upper, lower)
# 测试例子
input_string = "AAaaBBb123CCccccd"
result = count_letters(input_string)
print(result) # 输出: upper=6, lower=9
```
在这个函数中,`isupper()`和`islower()`分别用于判断字符是否为大写或小写字母,`sum(1 for ...)`会计算满足条件的字符数量。
C语言分别统计字符串中大写字母和小写字母的个数。例如,输入字符串s:“AAaaBBb123CCccccd”,则应输出结果:upper=6,lower=3。
在C语言中,你可以通过遍历字符串并使用字符处理函数来统计大写字母和小写字母的数量。这里是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符类型的库函数
int main() {
char s[] = "AAaaBBb123CCccccd";
int upper_count = 0, lower_count = 0;
int i;
for (i = 0; s[i] != '\0'; i++) { // 遍历字符串直到遇到空字符'\0'
if (isupper(s[i])) { // 检查是否为大写字母
upper_count++;
} else if (islower(s[i])) { // 否则是小写字母
lower_count++;
}
}
printf("upper=%d, lower=%d\n", upper_count, lower_count);
return 0;
}
```
这个程序首先初始化两个计数器 `upper_count` 和 `lower_count`,然后逐个检查字符串中的每个字符。如果字符是大写字母,就增加 `upper_count`;如果是小写字母,则增加 `lower_count`。最后,它打印出大写字母和小写字母的总数量。
阅读全文
相关推荐
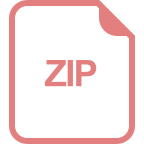
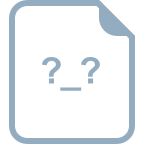
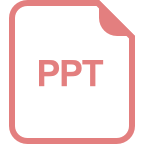
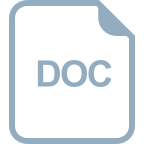
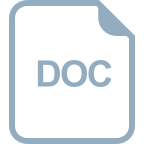
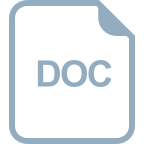
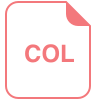


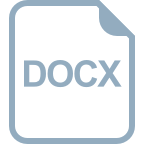
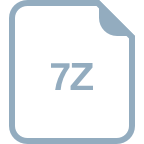
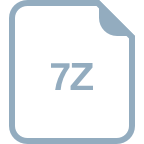
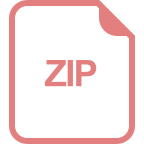