winform 输入查询条件在FTp查找并显示在gridcontrol中并可以下载文件
时间: 2024-11-09 16:17:26 浏览: 22
在Windows Forms应用中,实现这样的功能通常涉及到以下几个步骤:
1. **设置Ftp连接**:首先,你需要使用FTP库(如FluentFTP.NET、Net.FTPClient等)建立一个对FTP服务器的连接。在Winform中,你可以通过构造函数或配置属性设定用户名、密码和服务器地址。
```csharp
using FluentFTP;
FtpClient ftp = new FtpClient();
ftp.Connect("ftp.example.com", "username", "password");
```
2. **输入查询条件**:创建一个文本框或其他控件让用户输入他们想要搜索的关键字或文件名。用户输入后,保存到变量中以便后续使用。
```csharp
string searchTerm = textBoxSearch.Text;
```
3. **搜索文件**:编写一个方法来搜索FTP目录,根据用户的查询条件匹配文件名。这可能涉及遍历文件夹结构,或者直接使用FTP API的`FindFiles`方法。
```csharp
List<FtpFile> filesFound = ftp.FindFiles(searchTerm);
```
4. **显示结果**:将搜索结果添加到GridView中。对于每个找到的文件,你可以创建一个新的数据行,包含文件名、大小和其他相关信息。
```csharp
foreach (FtpFile file in filesFound)
{
dataGridView.Rows.Add(file.FullName, file.Size);
}
```
5. **下载按钮事件**:添加一个下载按钮,在点击时调用FTP客户端的`Download`方法下载选定的文件。通常会提供一个额外的选择确认过程,让用户选择下载的文件。
```csharp
private void buttonDownload_Click(object sender, EventArgs e)
{
if (dataGridView.SelectedRows.Count > 0)
{
string filePath = openFileDialog.FileName;
FtpFile selectedFile = filesFound[dataGridView.CurrentRow.Index];
ftp.Download(selectedFile.FullName, filePath);
MessageBox.Show("下载完成!");
}
}
```
6. **断开FTP连接**:在操作完成后,记得关闭FTP连接以释放资源。
```csharp
ftp.Disconnect();
```
阅读全文
相关推荐
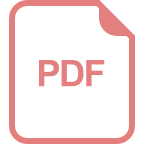
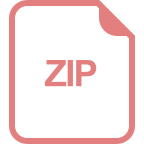
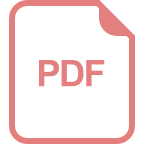
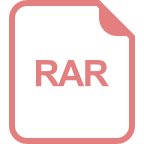
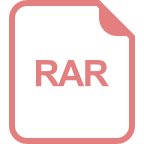
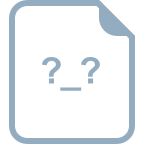
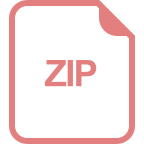
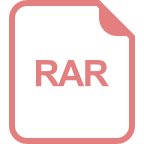
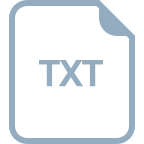
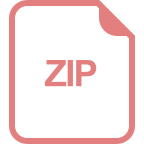
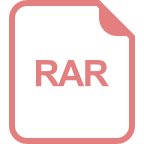
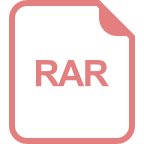
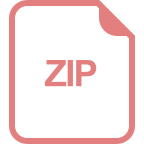
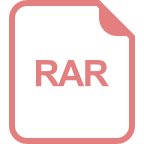
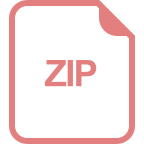
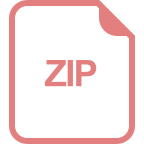
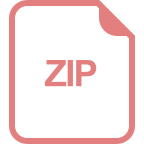
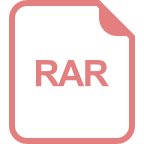