编写一个程序,模拟银行账户余额管理过程。初始余额设置为1000元。用户可以通过输入金额来模拟取款(输入正数)或存款(输入负数)操作,同时程序也允许用户通过输入'exit'来退出程序。
时间: 2024-12-16 08:22:25 浏览: 25
编写一个简单的银行账户余额管理程序,你可以用Python这样的脚本语言来实现。以下是一个基础的示例:
```python
class BankAccount:
def __init__(self, initial_balance=1000):
self.balance = initial_balance
def deposit(self, amount):
if amount > 0:
self.balance += amount
print(f"已成功存入{amount}元,当前余额为{self.balance}元")
else:
print("错误:存款金额必须是正数")
def withdraw(self, amount):
if amount < 0 and amount <= self.balance:
self.balance -= amount
print(f"已成功取出{-amount}元,当前余额为{self.balance}元")
elif amount >= self.balance:
print("错误:余额不足,无法完成取款操作")
else:
print("错误:取款金额必须是负数")
def exit_program(self):
print("感谢使用,再见!")
return True
# 创建银行账户实例并开始交互
account = BankAccount()
while not account.exit_program():
action = input("请输入操作(存款:deposit、取款:withdraw、退出:exit): ")
if action == 'deposit':
amount = float(input("请输入存款金额: "))
account.deposit(amount)
elif action == 'withdraw':
amount = float(input("请输入取款金额: "))
account.withdraw(amount)
elif action == 'exit':
continue
else:
print("无效的操作,请重新输入。")
```
在这个程序中,我们定义了一个`BankAccount`类,它有两个方法`deposit`和`withdraw`用于处理存款和取款,以及一个`exit_program`方法用于退出程序。用户可以通过交互式输入来选择操作和金额。
阅读全文
相关推荐





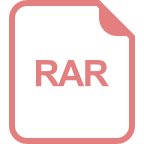









