校验wav文件格式c++
时间: 2023-08-16 07:07:50 浏览: 183
在C++中校验WAV文件格式,你可以按照以下步骤进行操作:
1. 打开WAV文件:使用C++中的文件操作函数打开WAV文件,确保成功打开文件。
2. 读取WAV文件头:从文件中读取WAV文件头信息,WAV文件头通常有44个字节,可以通过结构体来表示。
```cpp
#pragma pack(push, 1) // 确保以字节对齐方式读取结构体
struct WAVHeader {
char chunkID[4];
unsigned int chunkSize;
char format[4];
char subchunk1ID[4];
unsigned int subchunk1Size;
unsigned short audioFormat;
unsigned short numChannels;
unsigned int sampleRate;
unsigned int byteRate;
unsigned short blockAlign;
unsigned short bitsPerSample;
};
#pragma pack(pop)
```
3. 校验WAV文件头信息:根据WAV文件头的字段进行校验,例如检查chunkID是否为"RIFF",format是否为"WAVE",subchunk1ID是否为"fmt "等。
4. 检查音频格式:根据需要,可以进一步检查音频格式是否符合要求,例如检查音频采样率、声道数、位深度等。
5. 关闭文件:在校验完毕后,记得关闭文件。
下面是一个简单的示例代码,演示了如何校验WAV文件格式:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
bool validateWAVFile(const string& filename) {
ifstream file(filename, ios::binary);
if (!file) {
cout << "Failed to open file: " << filename << endl;
return false;
}
WAVHeader header;
file.read(reinterpret_cast<char*>(&header), sizeof(WAVHeader));
if (strncmp(header.chunkID, "RIFF", 4) != 0 ||
strncmp(header.format, "WAVE", 4) != 0 ||
strncmp(header.subchunk1ID, "fmt ", 4) != 0) {
cout << "Invalid WAV file format" << endl;
return false;
}
// 可以根据需要进一步校验其他字段
file.close();
return true;
}
int main() {
string filename = "example.wav";
bool isValid = validateWAVFile(filename);
if (isValid) {
cout << "WAV file format is valid" << endl;
} else {
cout << "WAV file format is invalid" << endl;
}
return 0;
}
```
请注意,此示例代码仅校验了WAV文件头信息,如果需要更详细的检查,你可以根据具体需求进一步扩展代码。
阅读全文
相关推荐
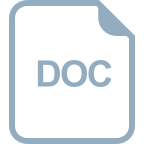
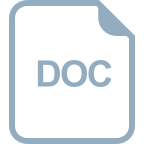
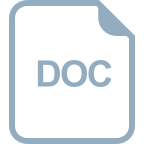
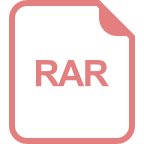
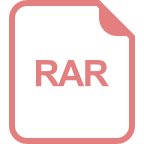
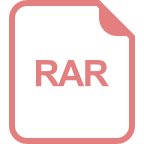
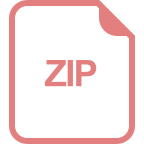
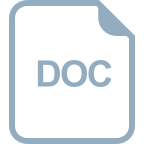
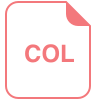
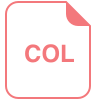
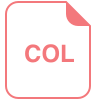
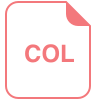
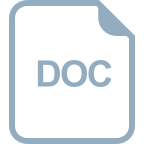
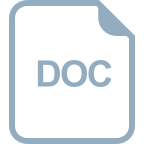
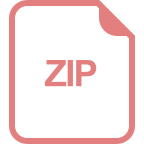
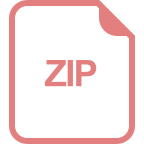
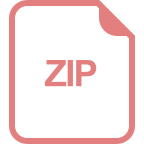
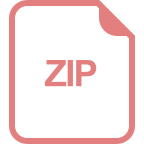
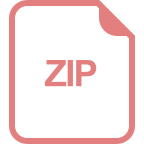