C++比较char类型字符相等
时间: 2023-09-06 10:10:33 浏览: 1051
在 C++ 中,可以使用等于运算符(==)来比较 char 类型的字符是否相等。例如:
```c++
char a = 'a';
char b = 'b';
if (a == b) {
// 如果 a 和 b 相等,执行这里的代码
} else {
// 如果 a 和 b 不相等,执行这里的代码
}
```
在上面的代码中,如果 a 和 b 的值相等,就会执行 if 语句中的代码块。否则,就会执行 else 语句中的代码块。
相关问题
c++比较字符串是否相等
在C++中,可以使用字符串比较函数`strcmp()`来比较两个字符串是否相等。`strcmp()`函数会比较两个字符串的每个字符,如果两个字符串完全一致,返回值为0。如果不一致,返回值会根据字符比较的结果而有所不同。
例如,以下代码可以比较两个字符串是否相等:
```c++
#include <iostream>
#include <cstring>
int main() {
char str1[] = "hello";
char str2[] = "world";
if (strcmp(str1, str2) == 0) {
std::cout << "The strings are equal." << std::endl;
} else {
std::cout << "The strings are not equal." << std::endl;
}
return 0;
}
```
在这个例子中,`strcmp()`函数将比较`str1`和`str2`两个字符串,由于它们不相等,所以输出结果为"The strings are not equal."
C++中如何比较字符是否相等
在C++中,可以使用运算符==来比较两个字符是否相等。例如:
```c++
char ch1 = 'a';
char ch2 = 'b';
if (ch1 == ch2) {
// ch1和ch2相等
} else {
// ch1和ch2不相等
}
```
注意,字符是用单引号括起来的,表示字符常量。当比较两个字符是否相等时,需要将它们放在运算符==的两侧。
另外,如果需要忽略大小写来比较两个字符是否相等,可以使用头文件<cctype>中的函数tolower()或toupper()将字符转换为小写或大写,然后再进行比较。例如:
```c++
#include <cctype>
char ch1 = 'A';
char ch2 = 'a';
if (tolower(ch1) == tolower(ch2)) {
// ch1和ch2相等(忽略大小写)
} else {
// ch1和ch2不相等(忽略大小写)
}
```
tolower()将大写字母转换为小写字母,toupper()将小写字母转换为大写字母。
相关推荐
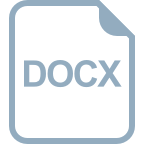
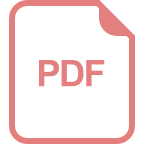
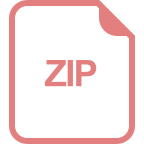












