请假代码java web_学生请假管理系统
时间: 2023-10-11 10:07:52 浏览: 171
以下是一个简单的学生请假管理系统的Java Web代码示例:
1. 创建一个名为 "StudentLeave" 的Java Web项目。
2. 在src目录下创建一个名为 "com.studentleave" 的包。
3. 在 "com.studentleave" 包中创建以下类:
- Student.java:学生实体类,包含学生姓名、学号、所在班级等信息。
```
package com.studentleave;
public class Student {
private String name;
private String id;
private String className;
public Student(String name, String id, String className) {
this.name = name;
this.id = id;
this.className = className;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className;
}
}
```
- LeaveApplication.java:请假申请实体类,包含请假学生、请假原因、请假开始时间、请假结束时间等信息。
```
package com.studentleave;
import java.util.Date;
public class LeaveApplication {
private Student student;
private String reason;
private Date startTime;
private Date endTime;
public LeaveApplication(Student student, String reason, Date startTime, Date endTime) {
this.student = student;
this.reason = reason;
this.startTime = startTime;
this.endTime = endTime;
}
public Student getStudent() {
return student;
}
public void setStudent(Student student) {
this.student = student;
}
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public Date getStartTime() {
return startTime;
}
public void setStartTime(Date startTime) {
this.startTime = startTime;
}
public Date getEndTime() {
return endTime;
}
public void setEndTime(Date endTime) {
this.endTime = endTime;
}
}
```
- LeaveApplicationDao.java:请假申请数据访问对象,用于对请假申请进行增删改查操作。
```
package com.studentleave;
import java.util.ArrayList;
import java.util.List;
public class LeaveApplicationDao {
private static List<LeaveApplication> leaveApplications = new ArrayList<>();
public void addLeaveApplication(LeaveApplication leaveApplication) {
leaveApplications.add(leaveApplication);
}
public void deleteLeaveApplication(LeaveApplication leaveApplication) {
leaveApplications.remove(leaveApplication);
}
public void updateLeaveApplication(LeaveApplication leaveApplication) {
int index = leaveApplications.indexOf(leaveApplication);
if (index != -1) {
leaveApplications.set(index, leaveApplication);
}
}
public List<LeaveApplication> getAllLeaveApplications() {
return leaveApplications;
}
}
```
4. 在WebContent目录下创建以下JSP页面:
- index.jsp:首页,包含一个表单用于提交请假申请。
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生请假管理系统</title>
</head>
<body>
<h1>学生请假管理系统</h1>
<form action="applyLeave.jsp" method="post">
<label for="name">姓名:</label>
<input type="text" id="name" name="name"><br><br>
<label for="id">学号:</label>
<input type="text" id="id" name="id"><br><br>
<label for="className">班级:</label>
<input type="text" id="className" name="className"><br><br>
<label for="reason">请假原因:</label>
<textarea id="reason" name="reason" rows="5" cols="40"></textarea><br><br>
<label for="startTime">开始时间:</label>
<input type="date" id="startTime" name="startTime"><br><br>
<label for="endTime">结束时间:</label>
<input type="date" id="endTime" name="endTime"><br><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
- applyLeave.jsp:处理请假申请,将申请信息存入数据库并显示申请成功信息。
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.util.Date" %>
<%@ page import="com.studentleave.*" %>
<%
String name = request.getParameter("name");
String id = request.getParameter("id");
String className = request.getParameter("className");
String reason = request.getParameter("reason");
Date startTime = java.sql.Date.valueOf(request.getParameter("startTime"));
Date endTime = java.sql.Date.valueOf(request.getParameter("endTime"));
Student student = new Student(name, id, className);
LeaveApplication leaveApplication = new LeaveApplication(student, reason, startTime, endTime);
LeaveApplicationDao dao = new LeaveApplicationDao();
dao.addLeaveApplication(leaveApplication);
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生请假管理系统</title>
</head>
<body>
<h1>学生请假管理系统</h1>
<p>请假申请已提交成功!</p>
<p>姓名:<%= name %></p>
<p>学号:<%= id %></p>
<p>班级:<%= className %></p>
<p>请假原因:<%= reason %></p>
<p>开始时间:<%= startTime %></p>
<p>结束时间:<%= endTime %></p>
</body>
</html>
```
- viewLeaves.jsp:查看所有请假申请。
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="com.studentleave.*" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生请假管理系统</title>
</head>
<body>
<h1>学生请假管理系统</h1>
<h2>所有请假申请</h2>
<table border="1">
<tr>
<th>姓名</th>
<th>学号</th>
<th>班级</th>
<th>请假原因</th>
<th>开始时间</th>
<th>结束时间</th>
</tr>
<%
LeaveApplicationDao dao = new LeaveApplicationDao();
List<LeaveApplication> leaveApplications = dao.getAllLeaveApplications();
for (LeaveApplication leaveApplication : leaveApplications) {
Student student = leaveApplication.getStudent();
%>
<tr>
<td><%= student.getName() %></td>
<td><%= student.getId() %></td>
<td><%= student.getClassName() %></td>
<td><%= leaveApplication.getReason() %></td>
<td><%= leaveApplication.getStartTime() %></td>
<td><%= leaveApplication.getEndTime() %></td>
</tr>
<% } %>
</table>
</body>
</html>
```
5. 在WebContent目录下创建以下Servlet:
- ViewLeavesServlet.java:处理查看所有请假申请的请求。
```
package com.studentleave;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/ViewLeavesServlet")
public class ViewLeavesServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.getRequestDispatcher("viewLeaves.jsp").forward(request, response);
}
}
```
6. 在web.xml配置文件中注册Servlet:
```
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
id="WebApp_ID" version="4.0">
<display-name>StudentLeave</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>ViewLeavesServlet</servlet-name>
<servlet-class>com.studentleave.ViewLeavesServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>ViewLeavesServlet</servlet-name>
<url-pattern>/viewLeaves</url-pattern>
</servlet-mapping>
</web-app>
```
7. 启动Tomcat服务器,访问 http://localhost:8080/StudentLeave/index.jsp 即可提交请假申请。访问 http://localhost:8080/StudentLeave/viewLeaves 查看所有请假申请。
阅读全文
相关推荐
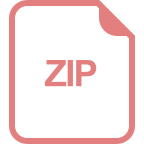
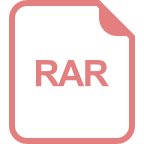
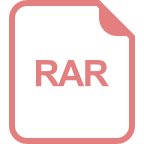
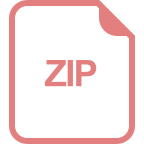
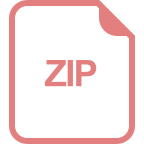
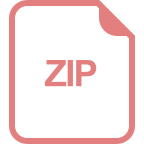
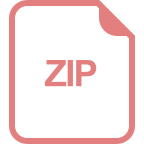
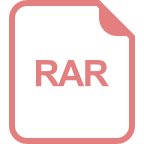
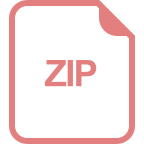
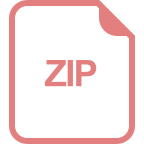
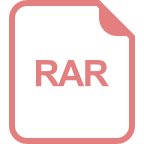
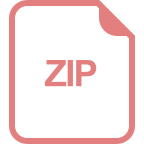
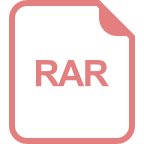

