java编写程序,要求从键盘输入若干个字符串(比如国家英文名称),并利用选择排序法将其升序后输出。
时间: 2024-05-05 10:16:14 浏览: 36
以下是Java程序代码:
```java
import java.util.Scanner;
public class SelectionSort {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入字符串个数:");
int n = scanner.nextInt();
String[] strs = new String[n];
for (int i = 0; i < n; i++) {
System.out.print("请输入第" + (i + 1) + "个字符串:");
strs[i] = scanner.next();
}
selectionSort(strs);
System.out.println("排序后的字符串为:");
for (String str : strs) {
System.out.println(str);
}
}
public static void selectionSort(String[] strs) {
int n = strs.length;
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (strs[j].compareTo(strs[minIndex]) < 0) {
minIndex = j;
}
}
if (minIndex != i) {
String temp = strs[i];
strs[i] = strs[minIndex];
strs[minIndex] = temp;
}
}
}
}
```
该程序首先从键盘输入字符串个数和具体字符串,然后调用`selectionSort`方法进行选择排序,最后输出排序后的字符串数组。`selectionSort`方法实现了选择排序的算法,通过遍历数组找到最小元素的下标,将其与当前位置的元素进行交换。程序使用`compareTo`方法进行字符串的比较,判断字符串的大小关系。
相关推荐
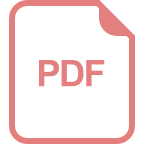
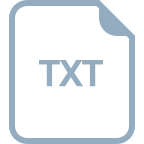














