Unity中使用Curvy用代码控制动画开始
时间: 2023-11-27 18:50:24 浏览: 98
要使用Curvy在Unity中控制动画开始,你需要在场景中先创建一个CurvySpline对象,并将其作为动画路径。你可以使用以下代码来控制动画的开始:
```csharp
using FluffyUnderware.Curvy;
public class AnimationController : MonoBehaviour
{
public CurvySpline spline; // reference to the CurvySpline object
public float speed = 1f; // animation speed
private float _progress = 0f;
private float _direction = 1f; // 1f for forward, -1f for backward
private Coroutine _animationCoroutine; // reference to the Coroutine used to start and stop the animation
private void Start()
{
// set the progress to 0f at the start
_progress = 0f;
}
public void StartAnimation()
{
// start the animation coroutine
if (_animationCoroutine == null)
_animationCoroutine = StartCoroutine(Animate());
}
public void StopAnimation()
{
// stop the animation coroutine
if (_animationCoroutine != null)
{
StopCoroutine(_animationCoroutine);
_animationCoroutine = null;
}
}
private IEnumerator Animate()
{
while (true)
{
// update the progress based on the speed and direction
_progress += speed * _direction * Time.deltaTime;
// check if the progress is out of bounds
if (_progress < 0f || _progress > 1f)
{
// reverse the direction if out of bounds
_direction *= -1f;
// clamp the progress back to bounds
if (_progress < 0f)
_progress = 0f;
else if (_progress > 1f)
_progress = 1f;
}
// update the position and rotation of the object along the spline
transform.position = spline.Interpolate(_progress);
transform.rotation = spline.GetOrientationFast(_progress);
yield return null;
}
}
}
```
在上述代码中,我们定义了一个AnimationController类,该类包含了一个CurvySpline对象的引用和一些控制动画的方法。在StartAnimation()方法中,我们启动了一个协程(coroutine),该协程不断更新动画的进度(progress),并根据进度在曲线上插值得到物体的位置和旋转,并在每一帧更新物体的Transform。在StopAnimation()方法中,我们停止了该协程。
你可以将该代码作为一个脚本组件添加到需要控制动画的物体上,然后在需要的时候调用StartAnimation()方法来启动动画。
阅读全文
相关推荐
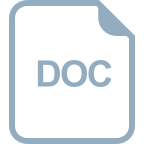
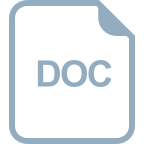
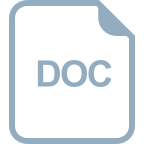

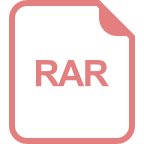
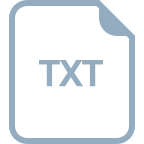
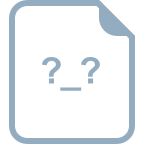
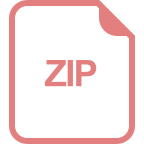
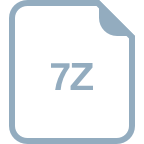
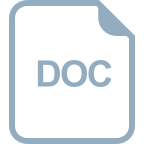
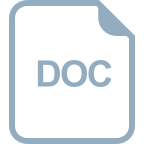
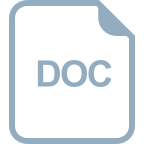
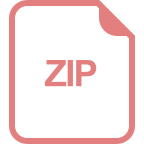
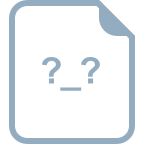
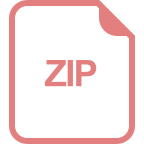
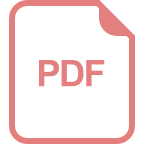
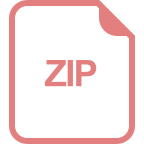
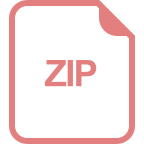