写一串单链表通讯录管理系统的代码
时间: 2023-11-29 08:05:41 浏览: 89
好的,以下是一个简单的单链表通讯录管理系统的代码示例,供您参考:
```python
class Contact:
def __init__(self, name, phone_number):
self.name = name
self.phone_number = phone_number
self.next = None
class ContactBook:
def __init__(self):
self.head = None
def add_contact(self, name, phone_number):
new_contact = Contact(name, phone_number)
if not self.head:
self.head = new_contact
else:
current_contact = self.head
while current_contact.next:
current_contact = current_contact.next
current_contact.next = new_contact
def delete_contact(self, name):
if not self.head:
print("Contact book is empty.")
elif self.head.name == name:
self.head = self.head.next
else:
current_contact = self.head
while current_contact.next and current_contact.next.name != name:
current_contact = current_contact.next
if not current_contact.next:
print(f"{name} not found in contact book.")
else:
current_contact.next = current_contact.next.next
def search_contact(self, name):
if not self.head:
print("Contact book is empty.")
else:
current_contact = self.head
while current_contact and current_contact.name != name:
current_contact = current_contact.next
if not current_contact:
print(f"{name} not found in contact book.")
else:
print(f"Name: {current_contact.name} Phone number: {current_contact.phone_number}")
def display_contacts(self):
if not self.head:
print("Contact book is empty.")
else:
current_contact = self.head
while current_contact:
print(f"Name: {current_contact.name} Phone number: {current_contact.phone_number}")
current_contact = current_contact.next
```
使用示例:
```python
contact_book = ContactBook()
# 添加联系人
contact_book.add_contact("Alice", "1234567890")
contact_book.add_contact("Bob", "2345678901")
contact_book.add_contact("Charlie", "3456789012")
# 显示所有联系人
contact_book.display_contacts()
# 查找联系人
contact_book.search_contact("Bob")
# 删除联系人
contact_book.delete_contact("Charlie")
# 显示所有联系人
contact_book.display_contacts()
```
希望这个示例代码可以帮助到您!
阅读全文
相关推荐
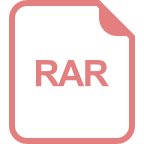















