springboot读取数据写入excel
时间: 2024-03-26 13:33:39 浏览: 132
在Spring Boot中,你可以使用Apache POI库来读取数据并将其写入Excel文件。下面是一个简单的示例代码:
1. 首先,确保在你的项目中添加了Apache POI的依赖。可以在pom.xml文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 创建一个ExcelUtil工具类,用于读取数据和写入Excel文件:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
public class ExcelUtil {
public static void writeDataToExcel(List<DataObject> dataList, String filePath) throws IOException {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Data");
int rowNum = 0;
for (DataObject data : dataList) {
Row row = sheet.createRow(rowNum++);
int colNum = 0;
row.createCell(colNum++).setCellValue(data.getId());
row.createCell(colNum++).setCellValue(data.getName());
row.createCell(colNum++).setCellValue(data.getValue());
}
FileOutputStream outputStream = new FileOutputStream(filePath);
workbook.write(outputStream);
workbook.close();
outputStream.close();
}
public static List<DataObject> readDataFromExcel(String filePath) throws IOException {
Workbook workbook = WorkbookFactory.create(new File(filePath));
Sheet sheet = workbook.getSheetAt(0);
List<DataObject> dataList = new ArrayList<>();
for (Row row : sheet) {
DataObject data = new DataObject();
data.setId(row.getCell(0).getStringCellValue());
data.setName(row.getCell(1).getStringCellValue());
data.setValue(row.getCell(2).getStringCellValue());
dataList.add(data);
}
workbook.close();
return dataList;
}
}
```
3. 创建一个DataObject类,用于存储数据:
```java
public class DataObject {
private String id;
private String name;
private String value;
// 省略构造函数、getter和setter方法
// ...
}
```
4. 在你的业务逻辑中,可以使用ExcelUtil类来读取数据和写入Excel文件:
```java
public class YourService {
public void processData() {
// 读取数据
List<DataObject> dataList = ExcelUtil.readDataFromExcel("path/to/input.xlsx");
// 处理数据...
// 写入Excel文件
try {
ExcelUtil.writeDataToExcel(dataList, "path/to/output.xlsx");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这样,你就可以使用Spring Boot读取数据并将其写入Excel文件了。
阅读全文
相关推荐
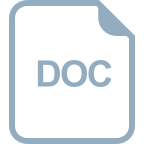
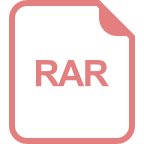
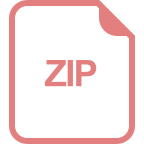
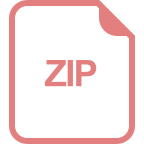
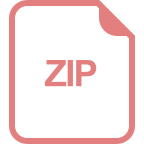
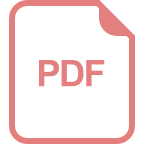
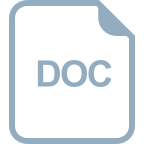
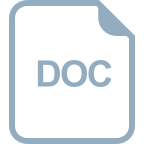







