请用java写出一个从压缩包里拿出指定文件并解压到指定位置的方法
时间: 2024-04-11 21:28:33 浏览: 23
当然可以,请参考以下的Java代码:
```java
import java.io.*;
import java.nio.file.*;
import java.util.zip.*;
public class UnzipUtil {
public static void unzipFile(String zipFilePath, String destFolderPath, String targetFileName) {
try {
File destFolder = new File(destFolderPath);
if (!destFolder.exists()) {
destFolder.mkdirs();
}
ZipInputStream zipInputStream = new ZipInputStream(new FileInputStream(zipFilePath));
ZipEntry zipEntry = zipInputStream.getNextEntry();
while (zipEntry != null) {
if (zipEntry.getName().equals(targetFileName)) {
String filePath = destFolderPath + File.separator + zipEntry.getName();
extractFile(zipInputStream, filePath);
break;
}
zipEntry = zipInputStream.getNextEntry();
}
zipInputStream.closeEntry();
zipInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private static void extractFile(ZipInputStream zipInputStream, String filePath) throws IOException {
BufferedOutputStream outputStream = new BufferedOutputStream(new FileOutputStream(filePath));
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = zipInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.flush();
outputStream.close();
}
public static void main(String[] args) {
String zipFilePath = "path/to/your/zip/file.zip";
String destFolderPath = "path/to/your/destination/folder";
String targetFileName = "file-to-extract.txt";
unzipFile(zipFilePath, destFolderPath, targetFileName);
}
}
```
你需要将 `zipFilePath` 替换为压缩包的实际路径,`destFolderPath` 替换为你想要解压到的目标文件夹的路径,`targetFileName` 替换为你要解压的文件名。
这个方法会遍历压缩包中的文件,当找到指定的文件后,将其解压到指定位置。
相关推荐
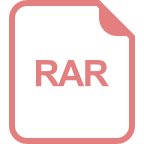
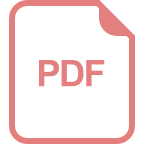
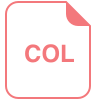













