JavaScript画带箭头的线
时间: 2023-07-20 15:27:44 浏览: 45
要画带箭头的线,可以借助 HTML5 的 Canvas 元素和 JavaScript。具体实现步骤如下:
1. 获取 Canvas 元素,并获取其上下文对象。
```
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
```
2. 设置线条样式
```
ctx.strokeStyle = "red"; // 设置线条颜色
ctx.lineWidth = 2; // 设置线条宽度
ctx.lineCap = "round"; // 设置线条末端样式
```
3. 开始绘制路径
```
ctx.beginPath(); // 开始路径
ctx.moveTo(x1, y1); // 移动到起始点
ctx.lineTo(x2, y2); // 绘制直线
```
4. 绘制箭头
```
var arrowSize = 10; // 设置箭头大小
var angle = Math.atan2(y2 - y1, x2 - x1); // 计算箭头角度
ctx.moveTo(x2, y2); // 移动到箭头点
ctx.lineTo(x2 - arrowSize * Math.cos(angle - Math.PI / 6), y2 - arrowSize * Math.sin(angle - Math.PI / 6)); // 绘制箭头线
ctx.moveTo(x2, y2);
ctx.lineTo(x2 - arrowSize * Math.cos(angle + Math.PI / 6), y2 - arrowSize * Math.sin(angle + Math.PI / 6));
```
5. 绘制线条
```
ctx.stroke(); // 绘制线条
```
完整代码示例:
```
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.strokeStyle = "red";
ctx.lineWidth = 2;
ctx.lineCap = "round";
ctx.beginPath();
ctx.moveTo(50, 50);
ctx.lineTo(200, 50);
var arrowSize = 10;
var angle = Math.atan2(0, 150);
ctx.moveTo(200, 50);
ctx.lineTo(200 - arrowSize * Math.cos(angle - Math.PI / 6), 50 - arrowSize * Math.sin(angle - Math.PI / 6));
ctx.moveTo(200, 50);
ctx.lineTo(200 - arrowSize * Math.cos(angle + Math.PI / 6), 50 - arrowSize * Math.sin(angle + Math.PI / 6));
ctx.stroke();
```
相关推荐
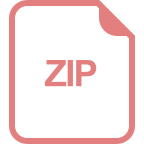
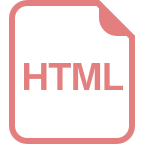
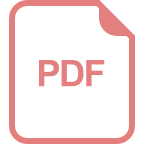














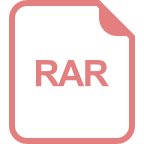