import os def del_files(path): for root,dirs,files in os.walk(path): for name in files: #print(name) if ".txt" in name: os.remove(os.path.join(root,name)) path=r'C:\iPLAS\Logs' del_files(path)
时间: 2024-04-28 07:27:14 浏览: 24
这段代码也是用 Python 语言编写的,主要是用来删除指定路径下的所有以“.txt”结尾的文件。首先,定义了一个名为 del_files 的函数,该函数接收一个路径参数 path。然后,使用 os.walk() 函数遍历这个路径下的所有目录和文件,对于每一个文件,如果文件名中包含“.txt”这个字符串,就使用 os.remove() 函数将这个文件删除。最后,调用 del_files() 函数并传入指定的路径参数 path,即可删除该路径下的所有以“.txt”结尾的文件。
相关问题
for root, dirs, files in os.walk(folder_path):
这段代码是用来遍历指定文件夹下的所有文件和子文件夹的。os.walk() 方法返回一个三元组 (root, dirs, files),其中:
- root:代表当前正在遍历的文件夹路径
- dirs:代表当前文件夹下的子文件夹列表
- files:代表当前文件夹下的文件列表
这个方法会遍历当前文件夹及其子文件夹,直到遍历完所有文件和文件夹为止。你可以使用这个方法来对文件夹下的所有文件进行遍历、查找、复制、移动等操作。
解释下面的代码: for root, dirs, files in os.walk(folder_path): for file_name in files:
这段代码使用Python中的os模块中的walk函数,用于遍历指定文件夹及其子文件夹中的所有文件和文件夹。os.walk会返回3个值,分别是当前遍历到的文件夹路径root、当前文件夹下的目录列表dirs、当前文件夹下的文件列表files。这个for循环中,我们只需要遍历所有的文件,因此针对files列表进行遍历,对于其中的每个文件名file_name,我们可以进行进一步的操作。
相关推荐
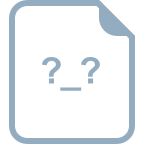
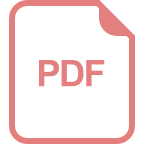
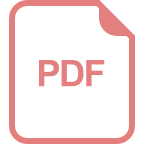













