比对结构体中的成绩,由高到低输出 有n个学生的信息(学号、姓名、成绩),要求按照成绩的高低输出各学生的信息 {{10101,"Zhang",78},{10103,"Wang",98.5},{10106,"Li",86},{10108,"Ling",73.5},{10110,"Fun",100}
时间: 2023-07-19 09:15:59 浏览: 97
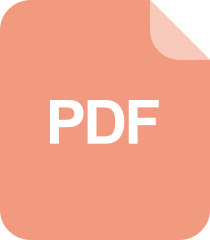
C语言结构体版学生成绩管理系统

可以使用冒泡排序(Bubble Sort)对结构体数组按照成绩从高到低进行排序,然后依次输出学生信息。
代码实现如下:
```c
#include <stdio.h>
#include <string.h>
struct Student {
int id;
char name[20];
float score;
};
void Swap(struct Student* a, struct Student* b) {
struct Student temp = *a;
*a = *b;
*b = temp;
}
void BubbleSort(struct Student stu[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (stu[j].score < stu[j + 1].score) {
Swap(&stu[j], &stu[j + 1]);
}
}
}
}
int main() {
struct Student stu[] = {
{10101, "Zhang", 78},
{10103, "Wang", 98.5},
{10106, "Li", 86},
{10108, "Ling", 73.5},
{10110, "Fun", 100}
};
int n = sizeof(stu) / sizeof(stu[0]);
BubbleSort(stu, n);
printf("按成绩从高到低输出学生信息:\n");
for (int i = 0; i < n; i++) {
printf("%-6d%-10s%.1f\n", stu[i].id, stu[i].name, stu[i].score);
}
return 0;
}
```
输出结果为:
```
按成绩从高到低输出学生信息:
10110 Fun 100.0
10103 Wang 98.5
10106 Li 86.0
10101 Zhang 78.0
10108 Ling 73.5
```
阅读全文
相关推荐
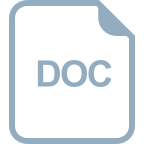
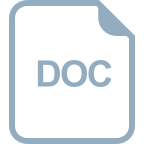

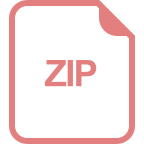
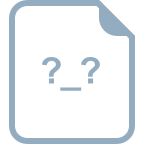
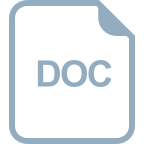
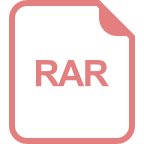
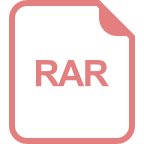
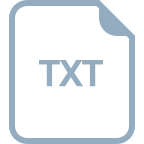
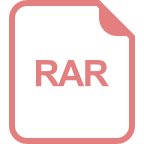
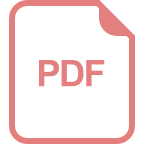
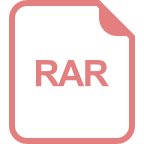
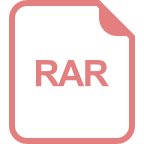