编写程序,使用Linux操作系统中的信号量机制模拟实现生产者-消费者问题。设有一个生产者和一个消费者,缓冲区可以存放产品,生产者不断生成产品放入缓冲区,消费者不断从缓冲区中取出产品,消费产品。
时间: 2024-05-13 15:14:47 浏览: 24
以下是使用Linux操作系统中的信号量机制模拟实现生产者-消费者问题的程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int in = 0;
int out = 0;
sem_t empty;
sem_t full;
sem_t mutex;
void *producer(void *arg) {
int item;
while (1) {
item = rand() % 100; // 生成一个随机数作为产品
sem_wait(&empty); // 等待缓冲区非满
sem_wait(&mutex); // 互斥访问缓冲区
buffer[in] = item; // 将产品放入缓冲区
in = (in + 1) % BUFFER_SIZE;
printf("Producer produced item %d\n", item);
sem_post(&mutex); // 释放缓冲区
sem_post(&full); // 增加缓冲区中的产品数量
sleep(1); // 生产者休眠1秒
}
pthread_exit(NULL);
}
void *consumer(void *arg) {
int item;
while (1) {
sem_wait(&full); // 等待缓冲区非空
sem_wait(&mutex); // 互斥访问缓冲区
item = buffer[out]; // 从缓冲区中取出产品
out = (out + 1) % BUFFER_SIZE;
printf("Consumer consumed item %d\n", item);
sem_post(&mutex); // 释放缓冲区
sem_post(&empty); // 减少缓冲区中的产品数量
sleep(2); // 消费者休眠2秒
}
pthread_exit(NULL);
}
int main() {
pthread_t tid_producer, tid_consumer;
sem_init(&empty, 0, BUFFER_SIZE); // 初始化信号量empty为BUFFER_SIZE
sem_init(&full, 0, 0); // 初始化信号量full为0
sem_init(&mutex, 0, 1); // 初始化信号量mutex为1
pthread_create(&tid_producer, NULL, producer, NULL);
pthread_create(&tid_consumer, NULL, consumer, NULL);
pthread_join(tid_producer, NULL);
pthread_join(tid_consumer, NULL);
sem_destroy(&empty);
sem_destroy(&full);
sem_destroy(&mutex);
return 0;
}
```
在上面的程序中,使用了3个信号量:empty、full、mutex。empty表示缓冲区的空闲空间,其初值为BUFFER_SIZE;full表示缓冲区中的产品数量,其初值为0;mutex用于实现对缓冲区的互斥访问,其初值为1。
生产者线程和消费者线程分别运行producer函数和consumer函数。在producer函数中,首先生成一个随机数作为产品,然后等待缓冲区非满,再互斥访问缓冲区,将产品放入缓冲区,最后释放缓冲区和增加缓冲区中的产品数量。在consumer函数中,首先等待缓冲区非空,然后互斥访问缓冲区,从缓冲区中取出产品,最后释放缓冲区和减少缓冲区中的产品数量。
在主函数中,创建生产者线程和消费者线程,等待它们运行结束后,销毁信号量。
相关推荐
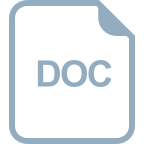
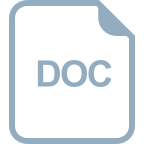
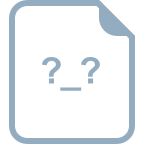





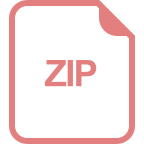
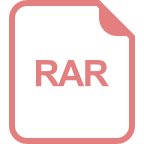
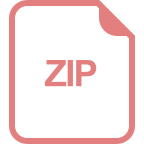
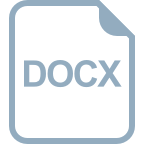
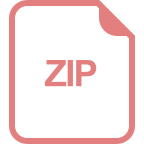
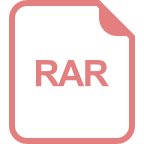
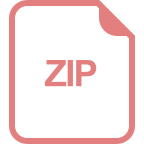
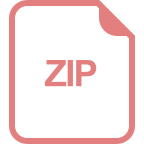
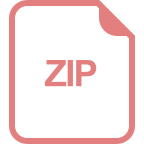
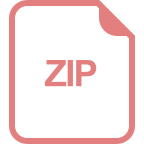