Uncaught TypeError: Cannot read properties of undefined (reading 'has')
时间: 2024-01-24 11:16:07 浏览: 121
Uncaught TypeError: Cannot read properties of undefined (reading 'has')错误通常发生在尝试访问一个未定义或空对象的属性时。这个错误的原因可能是你正在尝试访问一个不存在的属性,或者你的对象是空的。
解决这个错误的方法有以下几种:
1. 确保你正在访问的对象存在并且已经被正确初始化。你可以使用条件语句或者空值检查来避免访问未定义的属性。
2. 检查你的代码中是否有拼写错误或者语法错误。确保你正确地引用了对象和属性。
3. 如果你正在使用异步操作,比如Promise或者回调函数,确保你在访问对象属性之前已经获得了正确的数据。你可以使用条件语句或者异步操作的回调函数来处理这种情况。
4. 如果你使用的是框架或者库,比如Vue.js,检查你的模板或者组件是否正确地绑定了数据。确保你的数据在访问之前已经被正确地加载和初始化。
以下是一个示例代码,演示了如何避免Uncaught TypeError: Cannot read properties of undefined (reading 'has')错误:
```javascript
// 检查对象是否存在并且属性是否存在
if (myObject && myObject.property && myObject.property.has) {
// 执行操作
} else {
// 处理对象不存在或属性不存在的情况
}
```
相关问题
pinia Uncaught TypeError: Cannot read properties of undefined (reading 'has')
在你的问题中,报错信息是"Uncaught TypeError: Cannot read properties of undefined (reading 'has')"。这个错误通常发生在使用Pinia时,表示你尝试读取一个未定义的属性。这可能是因为你没有正确地初始化或使用Pinia。
解决这个问题的方法是确保正确地初始化和使用Pinia。以下是一些可能的解决方法:
1. 确保你已经正确地安装了Pinia,并在你的代码中导入了它。
2. 确保你已经正确地创建了Pinia实例,并将其绑定到Vue应用程序中。你可以在Vue应用程序的入口文件中进行这些操作。
3. 确保你在组件中正确地使用了Pinia。你可以通过在组件中导入Pinia实例并使用它来访问状态和操作。
4. 检查你的代码,确保没有尝试访问未定义的属性。你可以使用JavaScript的可选链操作符(?.)来避免访问未定义的属性。
以下是一个示例代码,演示了如何正确地使用Pinia:
```javascript
// 导入Pinia
import { createPinia } from 'pinia'
// 创建Pinia实例
const pinia = createPinia()
// 将Pinia实例绑定到Vue应用程序
app.use(pinia)
// 在组件中使用Pinia
export default {
setup() {
// 导入Pinia实例
import { useStore } from 'pinia'
// 创建store实例
const store = useStore()
// 访问状态和操作
console.log(store.someState)
store.someAction()
}
}
```
Uncaught TypeError: Cannot read properties of undefined (reading 'filter')
This error message indicates that you are trying to access the "filter" property of an undefined value. This can happen when you try to use the "filter" method on a variable that has not been properly initialized or defined.
To fix this error, you should check that the variable you are trying to use the "filter" method on is not undefined. If it is undefined, you will need to initialize it with the appropriate value before using the "filter" method.
For example, if you are trying to filter an array, you should check that the array is not undefined before using the "filter" method:
if (myArray !== undefined) {
// use the filter method on myArray
}
Alternatively, you can use a conditional statement to check if the variable is defined before using it:
if (typeof myVariable !== 'undefined') {
// use the filter method on myVariable
}
By checking that the variable is defined before using it, you can avoid the "Cannot read properties of undefined" error.
相关推荐
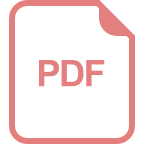
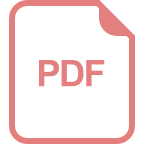
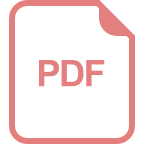










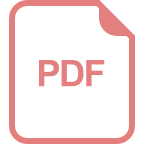
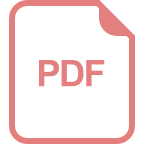
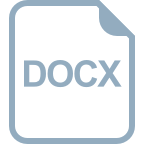