单项链表的就地逆转代码
时间: 2024-05-02 17:14:30 浏览: 96
单向链表的就地逆转是指在不创建新的链表节点的情况下,将原链表的节点顺序反转。下面是一个C语言实现的单向链表就地逆转的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 创建新节点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode == NULL) {
printf("内存分配失败\n");
exit(1);
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 将链表节点逆转
struct Node* reverseList(struct Node* head) {
struct Node* prev = NULL;
struct Node* current = head;
struct Node* next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
// 打印链表
void printList(struct Node* head) {
struct Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
// 创建链表
struct Node* head = createNode(1);
head->next = createNode(2);
head->next->next = createNode(3);
head->next->next->next = createNode(4);
head->next->next->next->next = createNode(5);
printf("原链表:");
printList(head);
// 将链表逆转
head = reverseList(head);
printf("逆转后的链表:");
printList(head);
return 0;
}
```
这段代码首先定义了一个链表节点的结构体,包含数据和指向下一个节点的指针。然后定义了创建新节点的函数`createNode`,用于创建新的链表节点。接下来是`reverseList`函数,用于将链表节点逆转。最后是`printList`函数,用于打印链表。
在`main`函数中,我们创建了一个包含5个节点的链表,并打印原链表。然后调用`reverseList`函数将链表逆转,并再次打印逆转后的链表。
运行以上代码,输出结果为:
```
原链表:1 2 3 4 5
逆转后的链表:5 4 3 2 1
```
阅读全文
相关推荐

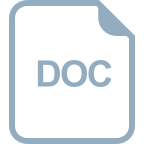










