利用结构体输入学生的学号、姓名、平时成绩、期末成绩
时间: 2023-05-31 18:17:59 浏览: 616
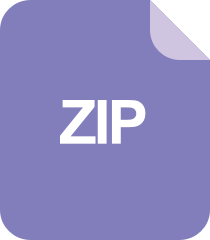
接收从键盘输入某班学生的学号、姓名、成绩,对学 生的成绩进行排序.zip

### 回答1:
可以定义一个包含学号、姓名、平时成绩和期末成绩的结构体,然后通过输入函数或者从文件中读取数据的方式,将学生的信息存储到结构体中。例如:
```
struct Student {
int id; // 学号
char name[20]; // 姓名
float score1; // 平时成绩
float score2; // 期末成绩
};
int main() {
struct Student stu;
printf("请输入学生的学号、姓名、平时成绩和期末成绩:\n");
scanf("%d %s %f %f", &stu.id, stu.name, &stu.score1, &stu.score2);
printf("学号:%d,姓名:%s,平时成绩:%f,期末成绩:%f\n", stu.id, stu.name, stu.score1, stu.score2);
return ;
}
```
这样就可以输入一个学生的信息,并将其存储到结构体中。如果需要输入多个学生的信息,可以使用循环语句来实现。
### 回答2:
在C语言中,结构体可以用来存储不同类型的数据,在这里我们使用结构体来存储学生的学号、姓名、平时成绩和期末成绩。以下是实现方法:
首先定义一个结构体,包含学号(ID)、名称(name)、平时成绩(score1)和期末成绩(score2):
struct student {
int ID;
char name[50];
float score1;
float score2;
};
然后,使用 scanf() 函数让用户输入学生的信息,如下所示:
struct student st;
printf("请输入学生学号:");
scanf("%d", &st.ID);
printf("请输入学生姓名:");
scanf("%s", st.name);
printf("请输入学生平时成绩:");
scanf("%f", &st.score1);
printf("请输入学生期末成绩:");
scanf("%f", &st.score2);
最后,输出学生的信息:
printf("学号:%d\n", st.ID);
printf("姓名:%s\n", st.name);
printf("平时成绩:%.2f\n", st.score1);
printf("期末成绩:%.2f\n", st.score2);
这样,我们就可以利用结构体输入学生的学号、姓名、平时成绩和期末成绩了。如果我们需要输入多个学生的信息,可以使用循环来实现,具体实现方法就不在此赘述。
### 回答3:
结构体是C语言中一种自定义的数据类型,它允许我们将不同类型的数据打包在一起,形成一个新的数据类型,从而更方便地操作数据。使用结构体可以非常方便地输入学生的学号、姓名、平时成绩和期末成绩。
首先,我们需要定义一个结构体,在这个结构体中包含学号、姓名、平时成绩和期末成绩这四个成员变量。我们可以这样定义一个名为Student的结构体:
```c
struct Student {
int number; // 学号
char name[20]; // 姓名
float score1; // 平时成绩
float score2; // 期末成绩
};
```
接下来,我们可以在程序中创建一个结构体变量,用来存储一个学生的信息。我们可以使用scanf函数来输入学生的学号、姓名、平时成绩和期末成绩。具体代码如下:
```c
#include <stdio.h>
struct Student {
int number; // 学号
char name[20]; // 姓名
float score1; // 平时成绩
float score2; // 期末成绩
};
int main()
{
struct Student stu;
printf("请输入学号: ");
scanf("%d", &stu.number);
printf("请输入姓名: ");
scanf("%s", stu.name);
printf("请输入平时成绩: ");
scanf("%f", &stu.score1);
printf("请输入期末成绩: ");
scanf("%f", &stu.score2);
printf("学号:%d,姓名:%s,平时成绩:%.2f,期末成绩:%.2f\n", stu.number, stu.name, stu.score1, stu.score2);
return 0;
}
```
在上面的代码中,我们定义了一个结构体变量stu,然后使用scanf函数输入学生的学号、姓名、平时成绩和期末成绩,并将输入的值分别赋给stu的成员变量number、name、score1和score2。最后,使用printf函数输出这个学生的信息。
需要注意的是,对于字符串类型的成员变量name,我们使用%s进行输入,而对于其他类型的成员变量,我们使用相应的格式字符进行输入。输入浮点数时,要使用%f格式字符,并使用&符号指定变量的地址。
通过以上的代码,我们可以方便地输入学生的学号、姓名、平时成绩和期末成绩,并且将这些信息存储在一个结构体变量中,方便以后的程序操作。
阅读全文
相关推荐
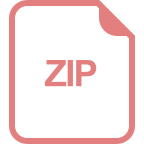
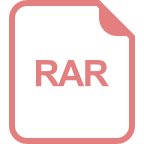









