unity 分割字符串
时间: 2023-09-13 11:08:06 浏览: 49
在 Unity 中,可以使用 C# 的 `Split()` 函数来分割字符串。以下是一个示例:
```csharp
string myString = "Hello,World";
string[] words = myString.Split(',');
foreach (string word in words) {
Debug.Log(word);
}
```
这段代码将字符串 `myString` 分割成两个单词,并使用逗号作为分隔符。`Split()` 函数返回一个字符串数组,其中包含分割后的单词。在上述示例中,`words` 数组将包含两个元素,分别为 `"Hello"` 和 `"World"`。然后可以使用 `foreach` 循环遍历这些单词,并对它们进行操作。
相关问题
unity字符串分割
在Unity中,你可以使用Split()方法来分割字符串。Split()方法接受一个字符数组作为参数,根据该字符数组中的字符将字符串分割成多个子字符串。以下是一个示例:
```csharp
string str = "Hello, World!";
string[] words = str.Split(' ');
foreach (string word in words)
{
Debug.Log(word);
}
```
上述示例中,我们使用空格字符作为分割符将字符串"Hello, World!"分割成两个子字符串"Hello,"和"World!",然后使用foreach循环遍历输出每个子字符串。
你可以根据自己的需求选择不同的分割符进行字符串分割。
unity 字符串截取
在Unity中,可以使用C#中的字符串截取函数来截取字符串。常用的字符串截取函数有Substring和Split。下面分别介绍这两个函数的用法。
1. Substring函数
Substring函数用于截取字符串的一部分。它的基本语法如下:
```
string str = "Hello, world!";
string subStr = str.Substring(startIndex, length);
```
其中,startIndex表示截取的起始位置,length表示截取的长度。例如,如果我们想截取字符串"Hello, world!"中的"world",可以这样写:
```
string str = "Hello, world!";
string subStr = str.Substring(7, 5);
```
这里的startIndex是7,表示从第8个字符开始截取;length是5,表示截取5个字符,即"world"。
2. Split函数
Split函数用于将字符串按照指定的分隔符分割成多个子串。它的基本语法如下:
```
string str = "apple,banana,orange";
string[] subStrs = str.Split(',');
```
其中,','表示分隔符,Split函数会将字符串按照','进行分割,得到三个子串"apple"、"banana"和"orange",并存储在一个字符串数组中。
在使用Split函数时,可以指定多个分隔符,例如:
```
string str = "apple,banana;orange";
string[] subStrs = str.Split(',', ';');
```
这里的分隔符是逗号和分号,Split函数会将字符串按照这两个分隔符进行分割,得到三个子串"apple"、"banana"和"orange"。
需要注意的是,Split函数返回的是一个字符串数组,每个元素是分割后的子串。如果要访问某个子串,可以通过数组的下标来获取,例如:
```
string str = "apple,banana,orange";
string[] subStrs = str.Split(',');
string subStr = subStrs[1]; // 获取第二个子串"banana"
```
相关推荐
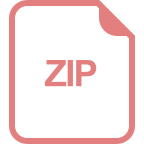












