app inventor调用图像识别_用App Inventor做一个人脸识别的app
时间: 2023-12-03 16:04:07 浏览: 108
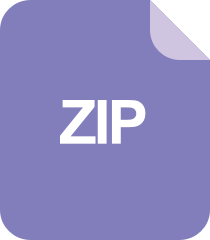
期末大作业——App Inventor 实现人脸识别等三个功能app大作业全套(包含课程设计说明书)(导入即可用)

使用 App Inventor 进行图像识别需要借助第三方 API,这里以使用百度人脸识别 API 为例。
1. 注册百度云账号并创建人脸识别应用,获取 API Key 和 Secret Key;
2. 在 App Inventor 中导入 Web 模块,配置 Web1 的属性 URL 为百度人脸识别 API 的请求地址,Method 为 POST;
3. 在 App Inventor 中导入 Camera 模块,配置 Camera1 的属性 Quality 为高;
4. 添加按钮组件,编写按钮 Click 事件处理程序,将 Camera1 拍摄的照片作为参数传递给 Web1 的 PostText 属性,同时在 Header 中设置 API Key 和 Secret Key;
5. 在 Web1 的 GotText 事件处理程序中解析百度人脸识别 API 的响应结果,获取人脸识别结果。
具体实现细节可以参考以下代码:
```
// 导入 Web 模块
import com.google.appinventor.components.runtime.Web;
// 导入 Camera 模块
import com.google.appinventor.components.runtime.Camera;
public class MainActivity extends AppCompatActivity {
// 声明组件
private Camera camera1;
private Web web1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化组件
camera1 = (Camera) findViewById(R.id.camera1);
web1 = (Web) findViewById(R.id.web1);
// 设置 Web1 的属性
web1.Url("https://aip.baidubce.com/rest/2.0/face/v3/detect");
web1.Method("POST");
web1.ContentType("application/json");
// 设置 Camera1 的属性
camera1.Quality(Camera.Quality_HIGH);
}
// 按钮 Click 事件处理程序
public void button1_Click(View view) {
// 获取 Camera1 的照片
Bitmap bitmap = camera1.getCameraImage();
// 将照片转换为 Base64 编码
ByteArrayOutputStream baos = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.JPEG, 100, baos);
byte[] bytes = baos.toByteArray();
String base64 = Base64.encodeToString(bytes, Base64.DEFAULT);
// 设置 Web1 的 Header
web1.Headers("Content-Type:application/json");
web1.Headers("Accept:application/json");
web1.Headers("Authorization:Bearer " + API_KEY);
// 设置 Web1 的 PostText 属性
web1.PostText("{\"image\":\"" + base64 + "\",\"image_type\":\"BASE64\",\"face_field\":\"age,gender,beauty,expression,face_shape,emotion\"}");
// 发送请求
web1.Go();
}
// Web1 的 GotText 事件处理程序
public void web1_GotText(String responseText) {
try {
// 解析响应结果
JSONObject jsonObject = new JSONObject(responseText);
JSONArray jsonArray = jsonObject.getJSONArray("result");
JSONObject resultObject = jsonArray.getJSONObject(0);
JSONObject faceObject = resultObject.getJSONObject("face_list").getJSONObject(0);
int age = faceObject.getInt("age");
String gender = faceObject.getString("gender");
double beauty = faceObject.getDouble("beauty");
String expression = faceObject.getJSONObject("expression").getString("type");
String faceShape = faceObject.getJSONObject("face_shape").getString("type");
String emotion = faceObject.getJSONObject("emotion").getString("type");
// 显示人脸识别结果
Toast.makeText(this, "年龄:" + age + "\n性别:" + gender + "\n颜值:" + beauty + "\n表情:" + expression + "\n脸型:" + faceShape + "\n情绪:" + emotion, Toast.LENGTH_LONG).show();
} catch (JSONException e) {
e.printStackTrace();
}
}
}
```
注意:这里只是一个简单的示例,实际应用中还需要考虑安全性、性能等问题。
阅读全文
相关推荐



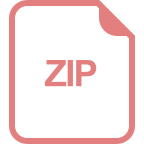
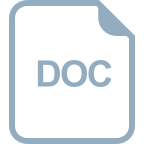
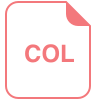
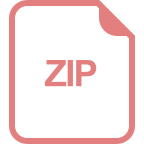
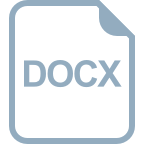
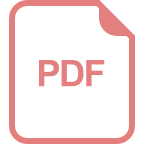
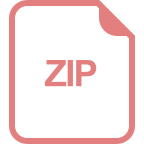