接口调用接收json报文
时间: 2023-08-10 08:58:30 浏览: 44
接口调用接收JSON报文的方法会根据你使用的编程语言和框架而有所不同。这里我以Python为例,给你一个简单的示例代码:
```python
import json
from flask import Flask, request
app = Flask(__name__)
@app.route('/api', methods=['POST'])
def api_handler():
# 获取POST请求中的JSON数据
data = request.get_json()
# 处理JSON数据
# TODO: 在这里根据需求进行相应的处理
# 返回响应
response = {'message': 'Success'}
return json.dumps(response), 200, {'Content-Type': 'application/json'}
if __name__ == '__main__':
app.run()
```
上述代码使用了Flask框架来创建一个简单的Web服务,当收到POST请求时,会从请求中获取JSON数据,并进行相应的处理。处理完成后,返回一个JSON格式的响应。
你可以根据自己的需求修改代码中的数据处理部分。同时,你也可以根据自己的开发环境和需求选择适合的编程语言和框架来进行接口调用和JSON报文的处理。
相关问题
vc ++ http接口调用 json
### 回答1:
vc是指Venture Capital(风险投资),是指投资者将资金投入于有潜力的初创企业或创新项目中,以获取高额回报的一种投资方式。vc不仅提供资金支持,还会向项目方提供战略指导、市场拓展、管理经验等方面的支持,帮助企业实现更快的发展。
在vc投资过程中,经常需要使用http接口调用json。HTTP是一种用于传输超文本的协议,而json是一种轻量级的数据交换格式。通过HTTP接口,投资者可以向初创企业的服务器发送请求,获取关于企业经营状况、财务数据、市场前景等信息,并将这些信息以json格式进行传输。
通过http接口调用json,vc投资者可以实时了解企业的运营情况和财务状况,以便做出更好的投资决策。同时,通过json可以将复杂的数据进行结构化处理,便于投资者进行数据分析和挖掘。投资者可以通过自己搭建的数据分析平台,将从http接口获取的json数据导入到系统中,进行数据处理和建模。这样,投资者可以更好地了解企业的市场定位、盈利模式、用户增长情况等,并根据这些数据进行风险评估和投资决策。
总之,vc投资者通过http接口调用json,可以实时获取企业的关键数据,在投资决策上提供更有针对性的支持。而企业也能通过向vc投资者提供http接口和json数据,展示自身的潜力和价值,从而吸引更多的投资。这种基于http接口调用json的交互方式,能够促进vc投资与初创企业之间的信息流动,进而推动创新与发展。
### 回答2:
VC(Voice Conversion,声音转换)是一种将一个人的语音转换为另一个人的语音的技术。在VC中,可以使用HTTP接口来进行JSON格式的调用。
HTTP接口调用是一种通过网络发送HTTP请求并接收HTTP响应的方式。JSON(JavaScript Object Notation,JavaScript对象表示法)是一种轻量级的数据交换格式,常用于前后端之间的数据传输。
在使用VC的HTTP接口调用JSON时,可以按照以下步骤进行:
1. 构建HTTP请求:使用程序或工具发送HTTP POST请求,指定接口的URL,并设置请求头部,告知服务器发送的数据格式是JSON。
2. 组织JSON数据:在请求体中,构建JSON数据,根据接口的要求填入相应的参数和数值。例如,可能需要指定要转换的语音文件路径、转换目标的人物特征等等。将这些信息以JSON格式组织起来。
3. 发送HTTP请求:将组织好的HTTP请求发送到服务器。服务器接收到请求后,会解析请求体中的JSON数据,并根据其中的参数进行相应的处理。
4. 处理请求:根据请求中包含的参数,服务器进行语音转换的计算和处理。可能涉及到语音信号处理、音素映射、声码器等复杂的算法和模型。
5. 返回JSON响应:服务器将处理结果组织成JSON格式的数据,并将其作为HTTP响应的内容返回给客户端。客户端接收到响应后,可以通过解析JSON数据来获得转换后的语音文件或其他相关信息。
通过VC的HTTP接口调用JSON,可以方便地实现语音转换的功能。这种方式可以让开发者使用自己熟悉的编程语言和工具进行开发,并且可以灵活地将语音转换集成到自己的应用程序中。
c# 调用接口返回json
可以使用 C# 的 HttpClient 和 Newtonsoft.Json 库来调用接口并解析 JSON 数据。
以下是一个简单的示例:
```csharp
using System;
using System.Net.Http;
using Newtonsoft.Json;
class Program
{
static async System.Threading.Tasks.Task Main(string[] args)
{
using (var httpClient = new HttpClient())
{
var response = await httpClient.GetAsync("https://example.com/api/data");
if (response.IsSuccessStatusCode)
{
var content = await response.Content.ReadAsStringAsync();
var data = JsonConvert.DeserializeObject<Data>(content);
Console.WriteLine($"Name: {data.Name}");
Console.WriteLine($"Age: {data.Age}");
}
else
{
Console.WriteLine($"Error: {response.StatusCode}");
}
}
}
}
class Data
{
public string Name { get; set; }
public int Age { get; set; }
}
```
在上面的示例中,我们使用 HttpClient 发送 GET 请求来获取 JSON 数据。如果响应成功,则使用 JsonConvert.DeserializeObject 方法将 JSON 数据转换为 C# 对象。最后,我们打印了获取到的数据的一些属性。
请注意,这只是一个简单的示例,实际情况可能更加复杂。例如,您可能需要在请求中设置标头,或者您可能需要使用 POST 请求发送数据。
相关推荐
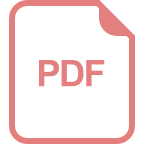











