要求:遍历文本文件words.txt中的所有行: (1)将‘baa’,‘babble’,‘babbler’,‘babe’,‘babel’,‘baboon’,‘baby’,‘babyhood’,‘Babylon’,‘Babylonian’ 单词添加到words.txt中单词后面 (2)统计文件总行数 (3)统计最长单词的长度 (4)统计首字母为大写A的单词 (5)将长度超过10的单词写入当前路径下的一个新文件
时间: 2023-07-24 13:39:11 浏览: 85
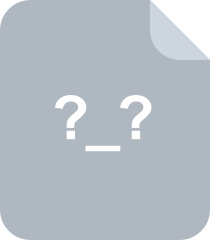
对txt文件中的单词进行计数
好的,下面是实现这些要求的 Python 代码:
```python
# (1) 将指定单词添加到 words.txt 文件中
words_to_add = ['baa', 'babble', 'babbler', 'babe', 'babel', 'baboon', 'baby', 'babyhood', 'Babylon', 'Babylonian']
with open('words.txt', 'a') as f:
for word in words_to_add:
f.write(word + '\n')
# (2) 统计文件总行数
with open('words.txt', 'r') as f:
line_count = 0
for line in f:
line_count += 1
print('文件总行数为:', line_count)
# (3) 统计最长单词的长度
with open('words.txt', 'r') as f:
max_length = 0
for line in f:
word = line.strip()
if len(word) > max_length:
max_length = len(word)
print('最长单词的长度为:', max_length)
# (4) 统计首字母为大写A的单词
with open('words.txt', 'r') as f:
count = 0
for line in f:
word = line.strip()
if word.startswith('A'):
count += 1
print('首字母为大写A的单词数为:', count)
# (5) 将长度超过10的单词写入新文件
with open('words.txt', 'r') as f, open('long_words.txt', 'w') as f_out:
for line in f:
word = line.strip()
if len(word) > 10:
f_out.write(word + '\n')
```
以上代码中,我们使用了 Python 内置的文件操作函数 `open` 来打开文件,然后使用 `for` 循环遍历每一行内容,进行相应的处理并输出结果。其中,文件打开模式使用的是 `'r'` 表示读取模式,使用的是 `'a'` 表示追加模式,使用的是 `'w'` 表示写入模式。
阅读全文
相关推荐




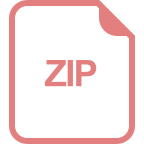
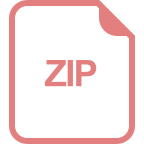
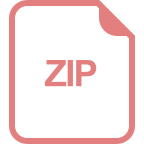
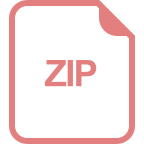
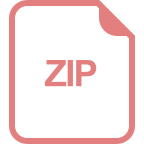
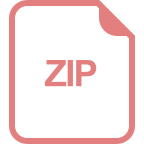
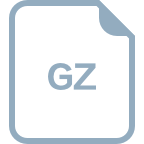
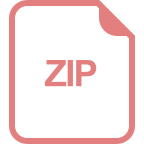
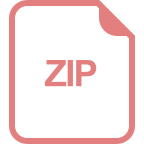
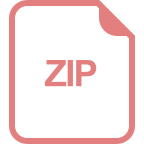
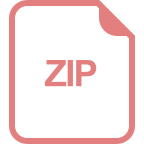
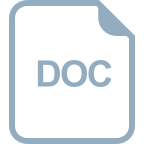
